牛顿迭代法解方程:y=x^2-2 c语言
时间: 2024-11-20 14:34:56 浏览: 37
牛顿迭代法是一种数值分析方法,用于近似求解非线性方程的根。在C语言中,对于函数f(x) = x^2 - 2,我们想要找到它的零点,即解决x^2 - 2 = 0。以下是使用牛顿迭代法的基本步骤:
1. 定义初始猜测值 `x0`。
2. 使用公式:`xn+1 = xn - f(xn) / f'(xn)`,其中 `xn` 是当前估计值,`xn+1` 是下一个更接近真实解的估计,`f'(xn)` 表示函数f关于x在点xn处的导数。
3. 当新的估算值与前一次的差别小于某个阈值(例如0.0001),或者达到最大迭代次数,算法停止。
下面是一个简单的C语言函数实现牛顿迭代法的例子:
```c
#include <stdio.h>
#include <math.h>
double f(double x) { return pow(x, 2) - 2; }
double df(double x) { return 2 * x; }
double newton_raphson(double x0, double epsilon, int max_iterations) {
int iteration = 0;
while (fabs(f(x0)) > epsilon && iteration < max_iterations) {
double x_next = x0 - f(x0) / df(x0);
x0 = x_next;
iteration++;
}
if (iteration == max_iterations) {
printf("Warning: Maximum iterations reached.\n");
}
return x_next;
}
int main() {
double initial_guess = 4.0; // 选择一个初始猜测值
double tolerance = 0.0001;
int max_iter = 100;
double solution = newton_raphson(initial_guess, tolerance, max_iter);
printf("Solution found after %d iterations: %.6lf\n", max_iter, solution);
return 0;
}
```
在这个程序中,`initial_guess`是你开始搜索的数值,`epsilon`是迭代终止的标准,`max_iterations`是最大允许的迭代次数。运行这个程序会输出方程的一个近似解。
阅读全文
相关推荐
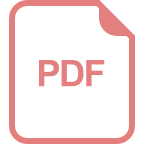
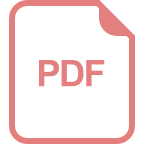







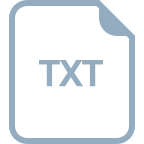
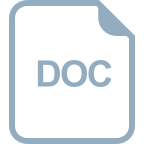
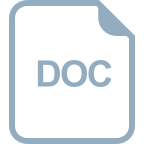




