用C语言代码生成分别用二分法和牛顿法解决非线性方程 x^5-2x^4+9x^3-9x^2+5x-9=0
时间: 2023-11-13 18:10:09 浏览: 116
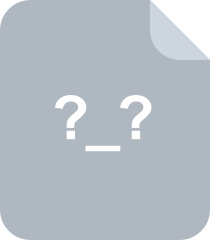
求解非线性方程牛顿法
以下是使用C语言实现二分法和牛顿法解决非线性方程 x^5-2x^4+9x^3-9x^2+5x-9=0 的示例代码:
```c
#include <stdio.h>
#include <math.h>
#define EPSILON 1e-6 // 精度
// 原方程
double f(double x) {
return pow(x, 5) - 2 * pow(x, 4) + 9 * pow(x, 3) - 9 * pow(x, 2) + 5 * x - 9;
}
// 二分法
double bisection(double a, double b) {
double c;
while ((b - a) > EPSILON) {
c = (a + b) / 2;
if (f(c) == 0.0)
return c;
else if (f(c) * f(a) < 0)
b = c;
else
a = c;
}
return c;
}
// 牛顿法
double newton(double x0) {
double x1 = x0 - f(x0) / (5 * pow(x0, 4) - 8 * pow(x0, 3) + 27 * pow(x0, 2) - 18 * x0 + 5);
while (fabs(x1 - x0) > EPSILON) {
x0 = x1;
x1 = x0 - f(x0) / (5 * pow(x0, 4) - 8 * pow(x0, 3) + 27 * pow(x0, 2) - 18 * x0 + 5);
}
return x1;
}
int main() {
double a = -2.0, b = 2.0, x0 = 1.0;
printf("二分法结果为:%lf\n", bisection(a, b));
printf("牛顿法结果为:%lf\n", newton(x0));
return 0;
}
```
在上述代码中,我们定义了两个函数 `bisection` 和 `newton` 分别实现二分法和牛顿法求解非线性方程。在 `main` 函数中,我们分别对方程 [-2, 2] 区间内的解进行了求解,并输出了结果。
需要注意的是,在实现牛顿法时,我们需要手动计算出原方程的导数,然后使用该导数进行迭代求解。这里直接给出了导数的表达式:f'(x) = 5x^4 - 8x^3 + 27x^2 - 18x + 5。
阅读全文
相关推荐
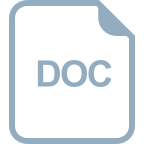
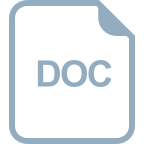
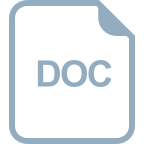

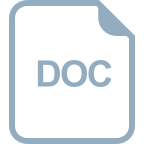
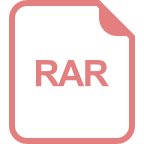
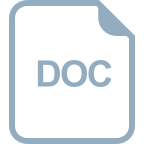
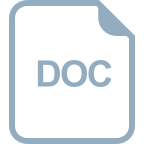
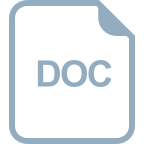
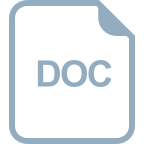
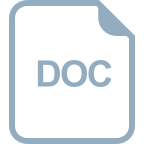
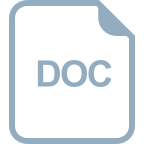



