用牛顿迭代法求f(x)=x*x-2的根 用C语言写
时间: 2024-10-11 08:02:39 浏览: 35
牛顿迭代法是一种常用的数值优化算法,用于寻找函数零点。对于方程 f(x) = x^2 - 2,我们可以使用它来逼近解。下面是使用C语言实现牛顿迭代法的一个简单示例:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return x * x - 2;
}
double df(double x) {
return 2 * x;
}
double newton_raphson(double initial_guess, double tolerance, int max_iterations) {
double x_new;
int i = 0;
if (f(initial_guess) == 0) {
printf("初始猜测值就是根\n");
return initial_guess;
}
x_new = initial_guess;
while (fabs(f(x_new)) > tolerance && i < max_iterations) {
x_new = x_new - f(x_new) / df(x_new);
i++;
}
if (i >= max_iterations) {
printf("达到最大迭代次数,未找到精确解\n");
}
return x_new;
}
int main() {
double guess = 1.0; // 初始猜测值
double tolerance = 1e-6; // 容许的误差范围
int max_iters = 100; // 最大迭代次数
double root = newton_raphson(guess, tolerance, max_iters);
printf("根的近似值: %.6lf\n", root);
return 0;
}
```
在这个程序中,`f()` 和 `df()` 函数分别计算函数值和导数。`newton_raphson()` 函数实现了牛顿迭代过程,直到满足给定的精度要求或达到最大迭代次数。在`main()` 函数里,我们设置了初始猜测值、容忍度和最大迭代次数,并输出了最终找到的根。
阅读全文
相关推荐
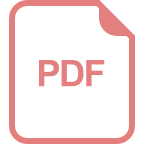
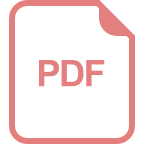
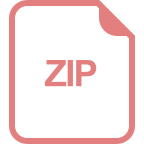















