编写程序将用户输入的阿拉伯数字转换为相应的中文大写数字
时间: 2024-11-09 08:29:56 浏览: 57
编写这样的程序通常需要一个包含对应数字及其中文名称的映射表。你可以使用循环或字典数据结构来存储这个转换规则。以下是一个简单的Python示例:
```python
def number_to_chinese(num):
chinese_digits = {
0: '零',
1: '一',
2: '二',
3: '三',
4: '四',
5: '五',
6: '六',
7: '七',
8: '八',
9: '九'
}
# 对于较大的数,可以继续拆分成单个位数并拼接中文
def helper(n):
if n < 10:
return chinese_digits[n]
else:
ten_place = n // 10
unit_place = n % 10
return chinese_digits[ten_place] + ('十' if ten_place > 1 else '') + (chinese_digits[unit_place] if unit_place != 0 else '')
result = ''
while num > 0:
result = helper(num % 10) + result
num //= 10
return result
# 示例使用
num_input = int(input("请输入一个阿拉伯数字:"))
print(f"对应的中文大写数字是:{number_to_chinese(num_input)}")
```
在这个程序中,我们首先定义了从0到9的数字对应的中文字符,然后定义了一个递归辅助函数`helper`来处理多位数。主函数会不断取余并将结果添加到前面,直到数字变为0。
阅读全文
相关推荐
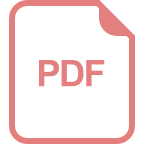
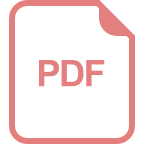


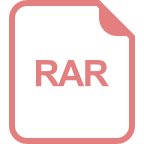
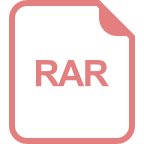



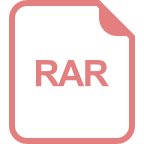
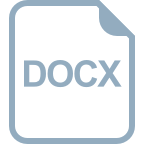
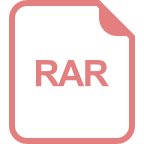
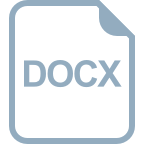
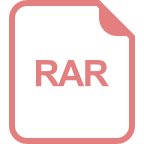



