nodejs实现留言板
时间: 2023-11-08 16:56:17 浏览: 91
要使用Node.js实现留言板功能,你可以按照以下步骤进行操作:
1. 创建一个index.html文件,用于显示留言板界面。
2. 在public目录中创建静态资源文件,用于处理用户的请求。当请求/public/xxx时,可以使用相应的处理函数来处理请求。
3. 使用引擎模块art-template来渲染页面。可以通过template.render()方法来解析页面,并获取返回的HTML字符串。
4. 使用POST请求来处理留言信息。在Express中,可以使用第三方包body-parser来解析表单POST请求体。需要先安装body-parser模块,然后使用app.use()来配置body-parser中间件,以解析请求体。然后通过app.post()来处理POST请求,并在回调函数中获取请求体的内容。
下面是一个示例代码:
```javascript
// index.html
<html>
<head>
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<form action="/post" method="post">
<label>姓名:</label>
<input type="text" name="name"><br>
<label>留言内容:</label>
<textarea name="message"></textarea><br>
<button type="submit">提交</button>
</form>
<div id="comments">
<!-- 这里会使用模板引擎来渲染留言内容 -->
</div>
</body>
</html>
// server.js
const express = require('express');
const template = require('art-template');
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
// 配置 body-parser 中间件
app.use(bodyParser.urlencoded({ extended: false }));
app.use(bodyParser.json());
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
app.post('/post', (req, res) => {
// 处理表单提交的留言信息
const name = req.body.name;
const message = req.body.message;
const date = new Date().toLocaleDateString();
// 这里使用模板引擎来渲染留言内容
const comments = [{ name: name, message: message, date: date }];
const html = template.render(__dirname + '/index.html', { comments });
res.send(html);
});
app.listen(port, function (error) {
if (error) return console.log('error!');
console.log('running....');
});
```
阅读全文
相关推荐


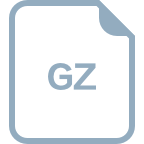















