points = np.genfromtxt('data.csv', delimiter=',')
时间: 2024-10-06 16:05:24 浏览: 25
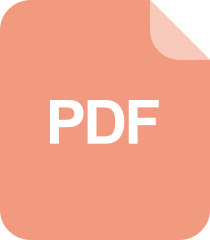
node.js中的path.delimiter方法使用说明
`np.genfromtxt` 是numpy库中的函数,用于从文本文件读取数据并转换成数组。当你使用 `points = np.genfromtxt('data.csv', delimiter=',')` 这一行时,它做了以下几件事[^2]:
1. **读取文件**: 它读取指定路径"data.csv"中的数据。
2. **分隔符设定**: "delimiter=','" 指定数据是以逗号(,)作为字段分隔符。
3. **返回数组**: 返回的数据被转化为一个二维数组(可能是表格形式),其中每一行代表一个观测记录,每一列表示一个变量。
具体操作步骤如下:
1. 导入所需的numpy模块:
```python
import numpy as np
```
2. 使用`genfromtxt`函数读取数据:
```python
points = np.genfromtxt('data.csv', delimiter=',')
```
3. 数据现在存储在名为 `points` 的变量中,你可以通过索引来访问其内容,如提取第0行和第0列的数据:
```python
x = points[0, 0] # 提取x坐标
y = points[0, 1] # 提取y坐标
```
4. 如果你想要可视化这些数据点,可以进一步使用matplotlib库绘制散点图,如前面提供的例子所示[^1]。
阅读全文
相关推荐
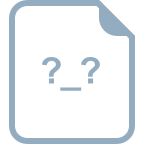

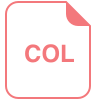




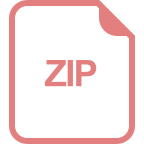
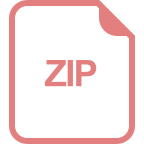
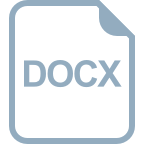
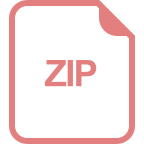
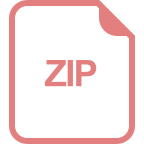
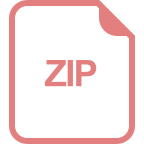
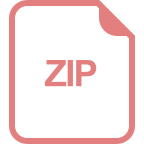
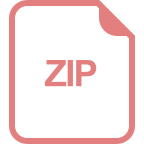