通过C语言函数实现线性查找算法
时间: 2024-11-13 22:25:05 浏览: 21
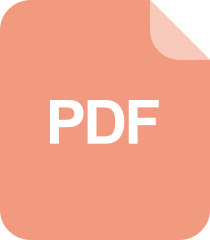
第7章 数组-5查找算法的函数实现1
线性查找,也称为顺序查找,是最基础的搜索算法之一。它的基本思想是从数组的第一个元素开始,逐个检查每个元素,直到找到目标元素或遍历完整个数组。下面是一个简单的C语言函数实现了线性查找:
```c
#include <stdio.h>
// 线性查找函数
int linearSearch(int arr[], int target, int n) {
// 遍历数组
for (int i = 0; i < n; i++) {
// 如果找到目标元素,则返回其索引
if (arr[i] == target) {
return i;
}
}
// 没有找到目标元素,返回-1表示元素不存在
return -1;
}
int main() {
int array[] = {1, 5, 8, 2, 6, 9};
int target = 6;
int size = sizeof(array) / sizeof(array[0]);
int index = linearSearch(array, target, size);
if (index != -1) {
printf("Element found at index: %d\n", index);
} else {
printf("Element not found in the array.\n");
}
return 0;
}
```
在上述代码中,`linearSearch` 函数接收一个整数数组、目标元素以及数组长度作为参数。它通过for循环依次比较每个元素,如果找到目标元素则立即返回该元素的索引,否则当遍历完数组仍未找到目标元素时,返回-1表示元素不存在于数组中。在主函数`main`中,我们定义了一个数组和目标值,然后调用线性查找函数并根据返回结果进行相应提示。
阅读全文
相关推荐
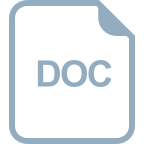
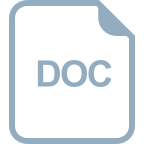
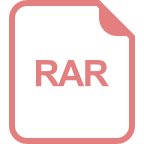
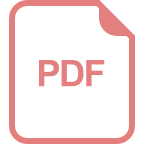
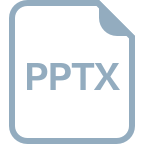
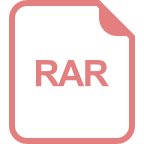
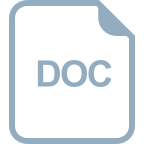
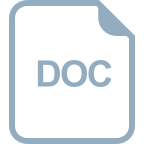
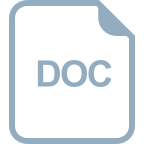
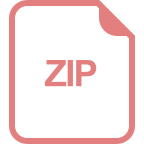
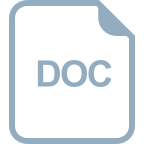
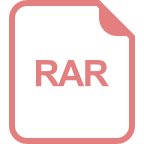
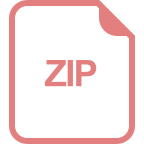
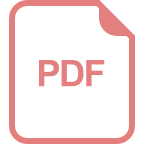
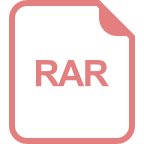
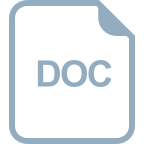
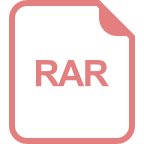
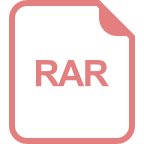