document.paragraphs
时间: 2023-08-30 13:07:31 浏览: 53
`document.paragraphs` 是 `python-docx` 库中用于访问 Word 文档中所有段落的属性。通过使用 `document.paragraphs`,你可以获取文档中的所有段落对象,并对它们进行迭代或执行其他操作。
以下是一个示例代码,演示了如何使用 `document.paragraphs` 属性来访问 Word 文档中的所有段落并打印它们的文本内容:
```python
from docx import Document
# 读取 Word 文档
document = Document("path/to/your/document.docx")
# 遍历文档中的所有段落
for paragraph in document.paragraphs:
print(paragraph.text)
```
在这个例子中,我们假设你已经将要读取的 Word 文档路径放在了 `"path/to/your/document.docx"` 中。你可以根据实际情况进行替换。
代码中的 `document.paragraphs` 返回一个包含文档中所有段落的列表。我们使用 `for` 循环遍历每个段落,并通过 `paragraph.text` 属性获取段落的文本内容。
希望这个示例能够帮助你!如果你还有其他问题,请随时提问。
相关问题
换一种写法,我的npoi版本不支持document.Paragraphs
如果你的NPOI版本不支持 `document.Paragraphs`,可以使用下面这种方式来实现Word文档的复制:
```
using (FileStream stream = new FileStream("path/to/your/document.docx", FileMode.Open, FileAccess.ReadWrite))
{
XWPFDocument document = new XWPFDocument(stream);
// 创建新的Word文档并将原始文档的内容复制到其中
XWPFDocument newDocument = new XWPFDocument();
foreach (IBodyElement element in document.BodyElements)
{
if (element.ElementType == BodyElementType.PARAGRAPH)
{
XWPFParagraph paragraph = (XWPFParagraph)element;
XWPFParagraph newParagraph = newDocument.CreateParagraph();
newParagraph.Alignment = paragraph.Alignment;
foreach (IRunElement run in paragraph.Runs)
{
if (run.ElementType == RunElement.TAB)
{
newParagraph.CreateRun().AddTab();
}
else if (run.ElementType == RunElement.TEXT)
{
XWPFRun newRun = newParagraph.CreateRun();
newRun.SetText(run.Text);
newRun.IsBold = run.IsBold;
newRun.IsItalic = run.IsItalic;
newRun.IsStrikeThrough = run.IsStrikeThrough;
newRun.IsUnderline = run.IsUnderline;
newRun.FontFamily = run.FontFamily;
newRun.FontSize = run.FontSize;
newRun.SetTextHighlightColor(run.GetHighlightedColor());
newRun.SetColor(run.GetColor());
}
}
}
else if (element.ElementType == BodyElementType.TABLE)
{
// 处理表格
}
}
// 将新文档保存
using (FileStream newStream = new FileStream("path/to/new/document.docx", FileMode.Create, FileAccess.ReadWrite))
{
newDocument.Write(newStream);
}
}
```
这种写法通过遍历文档中的 `BodyElements` 来获取段落和表格等元素,然后逐一复制到新文档中。需要注意的是,这种方法在处理表格时需要进行额外的处理,可以参考NPOI的官方文档进行实现。
为什么这串代码替换不了obj的内容。from openpyxl import load_workbook from docx import Document # 打开 Excel 文件 wb = load_workbook('data.xlsx') ws = wb.active # 打开 Word 模板文件 document = Document('template.docx') # 遍历 Excel 表格并填充 Word 模板 for row in ws.iter_rows(min_row=2, values_only=True): name, obj = row # 复制模板段落并插入数据 new_paragraph = document.add_paragraph() for run in document.paragraphs[0].runs: new_run = new_paragraph.add_run(run.text) if '{{name}}' in run.text: new_run.text = new_run.text.replace('{{name}}', name) elif '{{obj}}' in run.text: new_run.text = new_run.text.replace('{{obj}}', obj) # 保存 Word 文档 document.save('output.docx')
这段代码可以替换 `name` 的内容,但无法替换 `obj` 的内容是因为 `obj` 可能是一个元组或列表,而这些类型无法直接传递给 `new_run.text` 方法。解决方法是将 `obj` 转换为字符串,例如使用 `str()` 函数。修改后的代码如下:
```
from openpyxl import load_workbook
from docx import Document
# 打开 Excel 文件
wb = load_workbook('data.xlsx')
ws = wb.active
# 打开 Word 模板文件
document = Document('template.docx')
# 遍历 Excel 表格并填充 Word 模板
for row in ws.iter_rows(min_row=2, values_only=True):
name, obj = row
# 复制模板段落并插入数据
new_paragraph = document.add_paragraph()
for run in document.paragraphs[0].runs:
new_run = new_paragraph.add_run(run.text)
if '{{name}}' in run.text:
new_run.text = new_run.text.replace('{{name}}', name)
elif '{{obj}}' in run.text:
new_run.text = new_run.text.replace('{{obj}}', str(obj))
# 保存 Word 文档
document.save('output.docx')
```
相关推荐
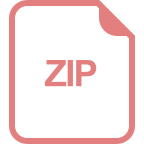
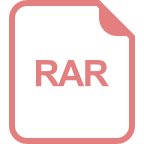
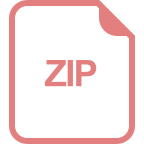













