unity使物体旋转
时间: 2023-09-10 11:12:15 浏览: 234
要在Unity中使物体旋转,你可以使用Transform组件的Rotate方法。这个方法接受一个Vector3参数,用于指定物体绕各个轴的旋转角度。
下面是一个简单的示例代码,展示如何使一个物体绕Y轴旋转:
```csharp
using UnityEngine;
public class RotateObject : MonoBehaviour
{
public float rotationSpeed = 10f; // 旋转速度
void Update()
{
// 获取当前物体的Transform组件
Transform transform = GetComponent<Transform>();
// 计算旋转角度
float rotationAmount = rotationSpeed * Time.deltaTime;
// 使用Rotate方法实现旋转
transform.Rotate(new Vector3(0, rotationAmount, 0));
}
}
```
将这个脚本附加到你想要旋转的物体上,然后调整rotationSpeed参数以控制旋转速度。在每一帧的Update方法中,物体将会绕Y轴旋转指定的角度。你也可以调整Rotate方法中的Vector3参数以实现不同轴向的旋转。
希望这能帮到你!
相关问题
unity 使物体旋转过渡
在Unity中,要创建物体旋转的平滑过渡效果,通常会使用“Animate”功能或者编写脚本来控制Transform组件。以下是简单的步骤:
1. **动画关键帧**:
- 首先,在Hierarchy视图中选中你要旋转的GameObject。
- 点击Inspector面板的"Add Component"添加Animation(动画)或Animator(动画控制器)组件。
- 创建一个新的旋转动画,添加两个关键帧:一个是初始静止位置,另一个是目标旋转角度。
- 调整时间轴上的关键帧插值,如设置从“Ease In”到“Ease Out”,使得旋转过程更平滑。
2. **C#脚本**:
- 使用C#编写一个Update或 LateUpdate函数,随着时间改变物体的旋转。
- 例如,可以使用`transform.Rotate()`方法,传入速度、持续时间和时间曲线(e.g., Quaternion.Lerp 或 Mathf.Lerp),逐渐改变物体的方向。
```csharp
float rotationSpeed = 10f; // 角度每秒的变化量
float transitionTime = 2f; // 旋转过渡的总时间
void Update()
{
float timeElapsed = Time.deltaTime;
transform.rotation = Quaternion.Lerp(transform.rotation, desiredRotation, timeElapsed / transitionTime);
}
```
unity 围绕物体旋转
可以使用 Transform.RotateAround 方法来实现围绕物体旋转。该方法需要传入三个参数:旋转中心点的坐标、旋转轴的方向和旋转的角度。
例如,如果要让一个物体以另一个物体为中心点绕着 Y 轴旋转 30 度,可以这样写:
```csharp
public Transform center; // 中心点物体的 Transform 组件
public float speed = 10f; // 旋转速度
void Update()
{
transform.RotateAround(center.position, Vector3.up, speed * Time.deltaTime);
}
```
其中,`center.position` 表示中心点物体的位置,`Vector3.up` 表示绕 Y 轴旋转,`speed * Time.deltaTime` 表示每帧旋转的角度。
阅读全文
相关推荐
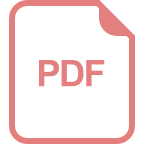
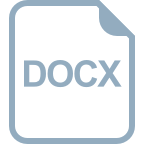
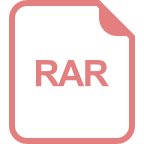






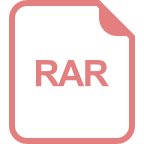
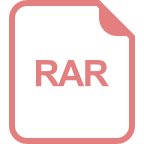
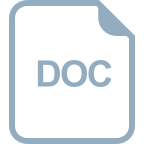
