java已知班级10名学生选修课课程的数字,语文,英语成绩,要求统计每门课的最高分,最低分,平均分及不及格的人数。
时间: 2024-10-16 17:18:51 浏览: 42
在Java中,为了统计每门课程的最高分、最低分、平均分以及不及格的人数,你可以创建一个包含所有学生信息的类,例如`Student`,并维护每个学生的课程分数。然后可以创建另一个类,比如`CourseStatistics`,用于计算课程统计数据。
首先,定义`Student`类:
```java
public class Student {
private String name;
private int[] scores = {0, 0, 0}; // 学生的语文、数学、英语成绩
public Student(String name) {
this.name = name;
}
// 添加获取和设置分数的方法...
}
```
接着,创建`CourseStatistics`类:
```java
import java.util.ArrayList;
import java.util.List;
public class CourseStatistics {
private List<Student> students;
public CourseStatistics(List<Student> students) {
this.students = students;
}
public void calculateScores() {
int totalStudents = students.size();
int maxScore[3] = new int[3], minScore[3] = new int[3];
int failedCount[3] = new int[3]; // 不及格人数数组
for (Student student : students) {
for (int i = 0; i < 3; i++) {
maxScore[i] = Math.max(maxScore[i], student.scores[i]);
minScore[i] = Math.min(minScore[i], student.scores[i]);
if (student.scores[i] < 60) { // 假设不及格线为60分
failedCount[i]++;
}
}
}
double averageScore[3] = new double[3];
for (int i = 0; i < 3; i++) {
averageScore[i] = (double)(totalStudents * maxScore[i]) / totalStudents;
}
System.out.println("语文:");
printStats(0, maxScore[0], minScore[0], averageScore[0], failedCount[0]);
System.out.println("数学:");
printStats(1, maxScore[1], minScore[1], averageScore[1], failedCount[1]);
System.out.println("英语:");
printStats(2, maxScore[2], minScore[2], averageScore[2], failedCount[2]);
}
private void printStats(int index, int max, int min, double avg, int failed) {
System.out.printf("最高分:%d,最低分:%d,平均分:%.2f,不及格人数:%d\n", max, min, avg, failed);
}
}
```
最后,创建一个示例的学生列表,并调用`calculateScores`方法:
```java
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
// 创建学生实例,添加成绩到students列表...
CourseStatistics courseStats = new CourseStatistics(students);
courseStats.calculateScores();
}
```
记得填充学生列表的部分,根据实际的10名学生的成绩数据构造`Student`对象。
阅读全文
相关推荐










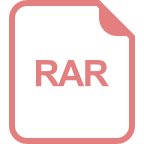






