模拟剪刀、石头和纸游戏,用s表示剪刀,r表示石头,p表示纸。游戏规则为剪刀剪纸,石头砸剪刀,纸包石头。两名游戏者分别输入s、r或p,按照游戏规则输出结果
时间: 2024-09-19 15:01:18 浏览: 36
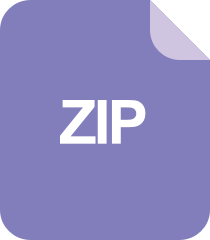
课程大作业基于python开发的剪刀石头布游戏猜拳游戏源码.zip
要模拟剪刀、石头、纸游戏,你可以创建一个简单的C++程序,让用户输入他们的选择(s、r或p),然后根据游戏规则决定胜负。以下是一个基本的控制台程序示例:
```cpp
#include <iostream>
#include <string>
// 定义游戏选项和对应的比较关系
enum class Move {
SCISSORS = 0, // s
ROCKS = 1, // r
PAPER = 2 // p
};
// 检查并返回胜者
Move determineWinner(const Move& move1, const Move& move2) {
if (move1 == move2) {
return Move::TIE;
} else if ((move1 == Move::SCISSORS && move2 == Move::PAPER) ||
(move1 == Move::ROCKS && move2 == Move::SCISSORS) ||
(move1 == Move::PAPER && move2 == Move::ROCKS)) {
return move1; // 第一个玩家获胜
} else {
return move2; // 第二个玩家获胜
}
}
int main() {
std::cout << "Enter your choice (s for scissors, r for rocks, p for paper): ";
char userChoice;
std::cin >> userChoice;
switch (userChoice) {
case 's':
userChoice = static_cast<char>(Move::SCISSORS);
break;
case 'r':
userChoice = static_cast<char>(Move::ROCKS);
break;
case 'p':
userChoice = static_cast<char>(Move::PAPER);
break;
default:
std::cerr << "Invalid input! Please choose s, r or p.\n";
return 1;
}
// 假设电脑随机选择
Move computerChoice = (rand() % 3) + Move::SCISSORS; // 随机生成0-2,对应scissors, rocks, paper
Move result = determineWinner(userChoice, computerChoice);
if (result == Move::TIE) {
std::cout << "It's a tie!\n";
} else {
std::cout << "You chose " << userChoice << ", and the computer chose "
<< computerChoice << ". You win!" << (result == userChoice ? "" : " The computer wins.\n");
}
return 0;
}
```
在这个程序中,我们首先定义了三种可能的选择和它们之间的比较关系。然后在`main()`函数中获取用户的输入,并将其转换成`Move`枚举值。接着,我们假设电脑随机选择一个选项。使用`determineWinner()`函数计算比赛结果,并显示给用户。
运行这个程序后,用户可以输入`s`, `r`, 或 `p`,程序会自动判断胜负。
阅读全文
相关推荐
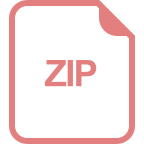
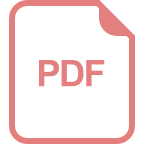
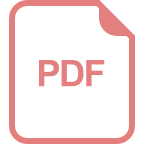
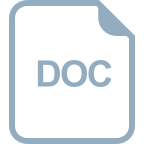
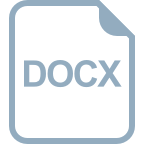
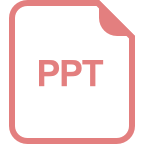

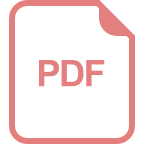
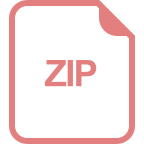
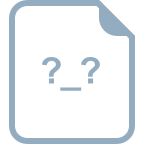
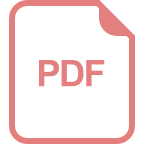
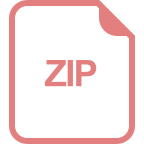
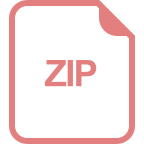
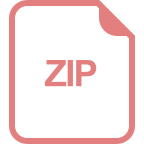
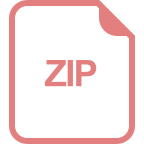
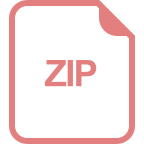