创建两个线程,要求如下: (1)一个线程输出100个1~26,另一个线程输出100个A~Z。 (2)一个线程使用集成Thread 类的写法,另一个线程使用实现Runnable接口的写法。 输出格式:
时间: 2024-12-25 14:28:06 浏览: 0
创建这样的多线程程序,我们可以分别使用集成`Thread`类的方式以及实现`Runnable`接口的方式来构造两个线程。这里我会给出一个简单的示例:
**使用集成`Thread`类的例子:**
```java
public class PrintNumbersAndLetters {
public static void main(String[] args) {
Thread numberThread = new Thread(new NumberPrinter());
Thread letterThread = new Thread(new LetterPrinter());
// 启动线程
numberThread.start();
letterThread.start();
// 等待所有线程结束
try {
numberThread.join();
letterThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
static class NumberPrinter implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
System.out.print(i + " ");
}
}
}
static class LetterPrinter implements Runnable {
@Override
public void run() {
for (char c = 'A'; c <= 'Z'; c++) {
System.out.print(c + " ");
}
}
}
}
```
**使用实现`Runnable`接口的例子:**
```java
public class PrintNumbersAndLettersRunnable {
public static void main(String[] args) {
Thread numberThread = new Thread(new NumberPrinterTask());
Thread letterThread = new Thread(new LetterPrinterTask());
numberThread.start();
letterThread.start();
// 等待所有线程结束
try {
numberThread.join();
letterThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
static class NumberPrinterTask implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
System.out.print(i + " ");
}
}
}
static class LetterPrinterTask implements Runnable {
@Override
public void run() {
for (char c = 'A'; c <= 'Z'; c++) {
System.out.print(c + " ");
}
}
}
}
```
这两个例子都会按照题目要求交替打印数字和字母。每个线程都有自己的任务范围,并独立地在各自的循环中执行。
阅读全文
相关推荐
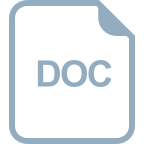
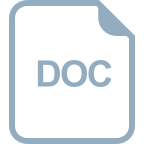
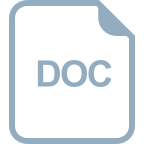
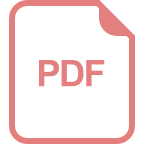
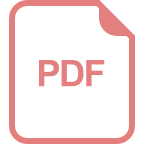
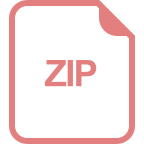
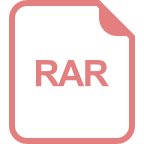
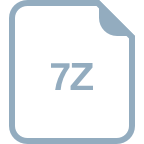
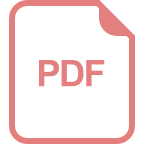
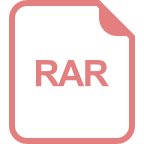
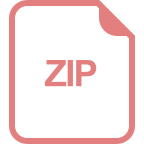
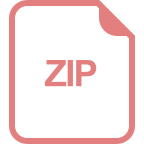
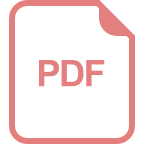
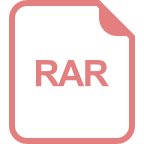
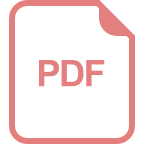
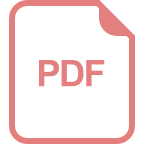
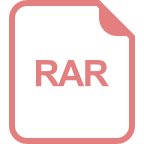
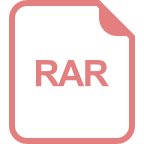