后端代码结构为controller、entity、enums、mapper、param、service,如何实现读取数据库中某一张表的信息并返回给前端一个json串?使用springboot架构,详细代码怎么写?
时间: 2024-09-09 11:10:55 浏览: 50
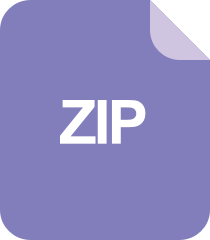
Java开发代码生成器:适配各种数据库、controller、entity、service、dao、mapper等可以自定义模板
在Spring Boot项目中,实现从数据库读取数据并以JSON格式返回给前端通常会包含以下几个步骤:
1. **Entity(实体类)**: 首先,你需要创建一个Java实体类(如`User.java`),代表数据库中的用户表。
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// 其他字段...
// getters and setters
}
```
2. **Repository(仓库接口)**: Spring Data JPA提供了一个名为`JpaRepository`的接口,你可以在此基础上创建一个自定义接口,如`CustomUserRepository.java`。
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface CustomUserRepository extends JpaRepository<User, Long> {
List<User> findAll();
User findById(Long id);
}
```
3. **Service(服务类)**: 在`UserService.java`中注入`UserRepository`,并实现获取所有用户信息的方法。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private CustomUserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
```
4. **Controller(控制器)**: 在`UserController.java`中接收请求,调用`UserService`并处理响应。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public ResponseEntity<List<User>> getUsers() {
List<User> users = userService.getAllUsers();
return ResponseEntity.ok(users); // 返回一个包含用户列表的HTTP响应
}
}
```
现在,当你访问`http://localhost:8080/users`,你会得到一个JSON格式的用户列表。
阅读全文
相关推荐
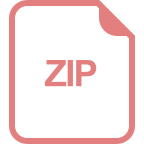
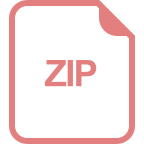

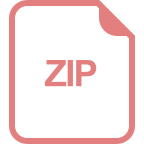
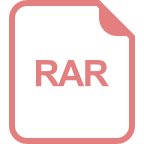
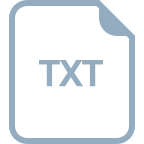
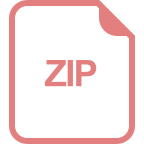
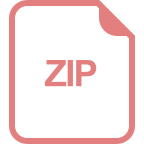
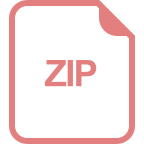
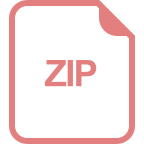
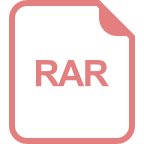
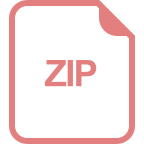
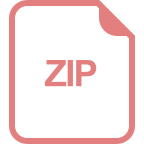
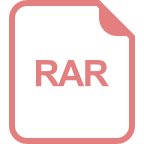
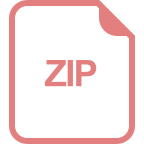
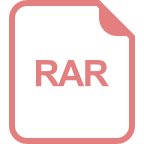
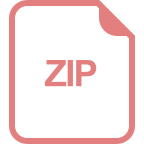