c++函数返回字符串
时间: 2024-12-17 07:55:51 浏览: 3
在 C++ 中,函数可以返回字符串,通常有两种方式:
1. **常量引用返回**:你可以通过返回 `const std::string&` 来返回一个字符串。这种方式不会复制整个字符串,而是返回一个指向内部存储的引用。这是最常见的做法,因为字符串通常是常量(不可修改),并且这样做节省了内存。
```cpp
std::string getHello() const {
return "Hello, World!";
}
```
2. **智能指针返回**:如果你想让返回的字符串可以在函数外部被安全地删除,可以使用 `std::unique_ptr<std::string>` 或 `std::shared_ptr<std::string>`。例如,如果你使用 `std::unique_ptr`:
```cpp
std::unique_ptr<std::string> createString() {
return std::make_unique<std::string>("Another string");
}
```
在这种情况下,一旦返回的指针离开其作用域,它所关联的字符串对象也会自动析构。
相关问题
C++函数返回字符串,返回值类型是char*
当你在C++中定义一个函数,其返回类型是`char*`,表示它会返回一个指向字符数组(即字符串)的指针。这样,在C++中创建字符串通常是通过动态内存分配实现的,因为`char*`本身只是一个地址,实际字符串的内容存储在别处。
下面是一个简单的示例,展示了如何创建和返回一个动态字符串:
```cpp
#include <cstring>
#include <cstdlib>
std::string* createString(const std::string& input) {
// 创建一个新的字符串,使用malloc动态分配内存
size_t len = input.length() + 1; // 加一是因为我们需要一个终止符'\0'
char* str = new char[len];
strcpy(str, input.c_str()); // 复制输入字符串到新分配的空间
// 返回指向新字符串的指针
return new std::string(str); // 注意这里返回的是一个指向std::string的指针,而不是直接的char*
}
```
要从C#中接收这个`char*`返回的字符串,正如你提到的,需要小心处理,因为C#默认不会自动解码这些字节。可以按照以下步骤操作:
1. 在C++中,当从DLL返回`char*`时,确保它已经被正确编码(通常UTF-8)。
2. C#端创建一个`byte[]`来接收字符串的字节序列,而不是直接尝试转换为`string`。
```csharp
[DllImport("your_dll.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern IntPtr GetString();
// 调用C++函数并获取结果
byte[] bytes = (byte[])Marshal.PtrToStructure(GetString(), typeof(byte[]));
// 使用Encoding.UTF8将字节数组转换回字符串
string result = Encoding.UTF8.GetString(bytes);
```
c++如何返回字符串数组
在 C++ 中,可以使用以下两种方式返回字符串数组:
1. 通过指针返回字符串数组
```c++
#include <iostream>
#include <string>
using namespace std;
// 返回字符串数组的函数
string* getStrings() {
static string strs[] = {"hello", "world", "how", "are", "you"};
return strs;
}
int main() {
// 调用函数获取字符串数组
string* strs = getStrings();
// 输出字符串数组
for (int i = 0; i < 5; i++) {
cout << strs[i] << endl;
}
return 0;
}
```
2. 通过引用参数返回字符串数组
```c++
#include <iostream>
#include <string>
using namespace std;
// 返回字符串数组的函数
void getStrings(string (&strs)[5]) {
strs[0] = "hello";
strs[1] = "world";
strs[2] = "how";
strs[3] = "are";
strs[4] = "you";
}
int main() {
// 定义字符串数组
string strs[5];
// 调用函数获取字符串数组
getStrings(strs);
// 输出字符串数组
for (int i = 0; i < 5; i++) {
cout << strs[i] << endl;
}
return 0;
}
```
注意:在第一种方式中,需要使用静态变量来存储字符串数组,否则在返回后该数组会被销毁。在第二种方式中,需要使用引用参数来传递字符串数组,否则仍然会复制整个数组,而不是返回指向数组的指针。
阅读全文
相关推荐
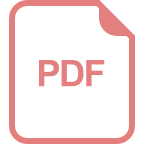
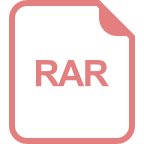
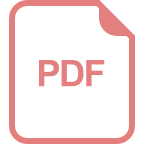













