C++字符串交换函数
时间: 2024-09-21 11:10:29 浏览: 40
C++中可以使用多种方法来交换两个字符串变量的内容。以下是几种常见的实现方式:
1. **逐字符赋值**:
```cpp
#include <string>
std::string swap(std::string& str1, std::string& str2) {
if (str1.length() != str2.length()) {
throw std::runtime_error("Strings must be of the same length.");
}
char temp;
for (size_t i = 0; i < str1.length(); ++i) {
temp = str1[i];
str1[i] = str2[i];
str2[i] = temp;
}
return str1;
}
```
2. **使用标准库算法**(C++11及以上版本):
```cpp
#include <algorithm>
std::string swap(std::string& str1, std::string& str2) {
if (str1.length() != str2.length()) {
throw std::runtime_error("Strings must be of the same length.");
}
str1.swap(str2);
return str2; // 返回的是被修改后的原始字符串
}
```
3. **模板函数**(通用性更强):
```cpp
template <typename T>
void swap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
// 调用时指定字符串类型
swap<std::string>(str1, str2);
```
阅读全文
相关推荐
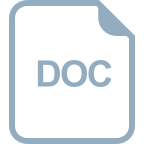
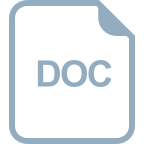
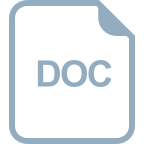
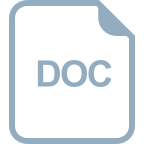

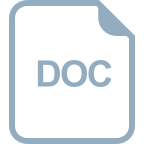
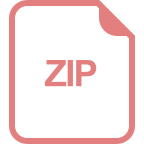
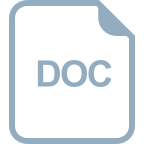
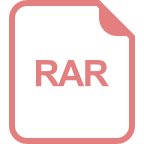
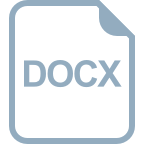
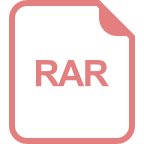
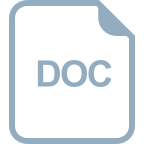
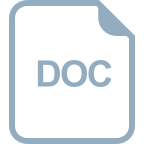
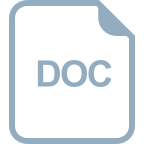




