c语言编写找出数组中第二大的数
时间: 2024-09-26 09:12:54 浏览: 73
在C语言中,找出数组中的第二大数可以通过遍历数组并维护两个变量来完成,一个是当前的最大值(max),另一个是次大值(second_max)。如果当前元素大于最大值,就将最大值赋给次大值,并更新最大值。下面是一个简单的示例:
```c
#include <stdio.h>
int findSecondLargest(int arr[], int size) {
// 初始化最大值和次大值为数组的第一个元素
int max = arr[0], second_max = arr[0];
// 遍历数组从第二个元素开始
for (int i = 1; i < size; i++) {
// 如果当前元素大于最大值,更新最大值和次大值
if (arr[i] > max) {
second_max = max;
max = arr[i];
// 否则,如果当前元素不是最大值但比次大值大,更新次大值
} else if (arr[i] > second_max && arr[i] != max) {
second_max = arr[i];
}
}
// 返回次大值
return second_max;
}
int main() {
int array[] = {5, 2, 8, 9, 1};
int size = sizeof(array) / sizeof(array[0]);
int second_largest = findSecondLargest(array, size);
printf("The second largest number is: %d\n", second_largest);
return 0;
}
```
这个程序会在给定数组中找到并返回第二大的数。如果数组中有相同的数值并且你想找到不同的第二大数(比如有三个相同的最大值),你需要稍作修改以忽略这些重复值。
阅读全文
相关推荐
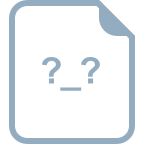
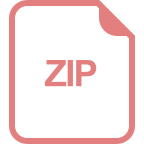
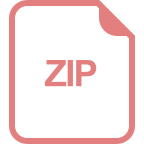





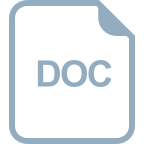
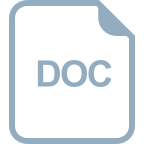








