vue2中使用高德地图,传入经纬度后,定位到指定位置,并显示传入的内容在地图上
时间: 2024-12-17 14:24:05 浏览: 8
在Vue2中使用高德地图(AMap),你可以通过以下几个步骤将传入的经纬度定位到地图上并显示相应内容:
1. 首先,你需要安装阿里的高德地图API库。可以使用npm命令安装:
```bash
npm install amap-jsapi-loader @vue-amap/core @vue-amap/map
```
2. 在`main.js`或其他入口文件中引入地图插件,并初始化地图:
```javascript
import Vue from 'vue'
import AMapCore from '@vue-amap/core'
import Map from '@vue-amap/map'
Vue.use(AMapCore)
Vue.component('amap-map', Map)
// 初始化地图,这里假设你已经获取了地图容器id
const map = new AMap.Map('mapContainer', {
zoom: 15, // 初始缩放级别
center: [116.404, 39.915], // 北京坐标作为默认中心点
});
```
3. 在组件中接收经纬度数据和需要展示的内容,然后创建标记(Marker):
```html
<template>
<div>
<amap-map :center="mapCenter" :zoom="zoomLevel">
<amap-marker v-for="(location, index) in locations" :position="location" :title="location.content">
<!-- 标记点击事件 -->
<template #popup>{{ location.content }}</template>
</amap-marker>
</amap-map>
</div>
</template>
<script>
export default {
data() {
return {
mapCenter: { lat: 0, lng: 0 }, // 初始地图中心
zoomLevel: 15,
locations: [
{ lat: yourLatitude, lng: yourLongitude, content: "示例内容1" },
// 添加更多地点...
],
};
},
};
</script>
```
4. 当你接收到新的经纬度数据时,更新`mapCenter`属性,地图会自动定位到新位置:
```javascript
methods: {
updateLocation(newLat, newLng, content) {
this.mapCenter = { lat: newLat, lng: newLng };
this.locations.push({ lat: newLat, lng: newLng, content });
}
}
// 使用时,在适当的地方调用updateLocation方法
```
阅读全文
相关推荐
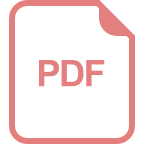
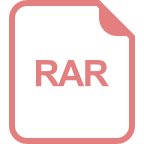
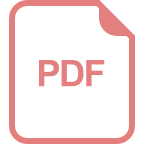
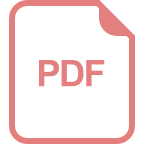
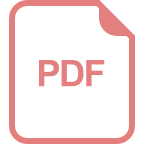
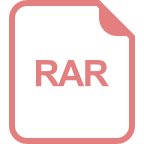
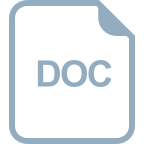











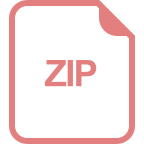