springboot整合redis 注解
时间: 2023-09-14 17:06:41 浏览: 109
在Spring Boot中,可以使用注解来实现与Redis的整合。首先,确保已经在pom.xml中添加了相应的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
接下来,需要配置Redis的连接信息,在application.properties或application.yml文件中添加以下配置:
```properties
spring.redis.host=your_redis_host
spring.redis.port=your_redis_port
```
然后,在需要使用Redis的类(比如Service或Repository)上添加`@EnableCaching`注解,以启用Spring Cache功能。
接下来,可以在需要使用Redis缓存的方法上使用`@Cacheable`、`@CachePut`、`@CacheEvict`等注解。
- `@Cacheable`:用于在方法执行前,先查询缓存,如果缓存中存在相应的数据,则直接返回;否则执行方法,并将方法的返回值存入缓存中。
- `@CachePut`:用于在方法执行后,将返回值存入缓存中。
- `@CacheEvict`:用于从缓存中移除相应的数据。
例如,下面是一个示例:
```java
@Service
@EnableCaching
public class UserService {
@Autowired
private UserRepository userRepository;
@Cacheable(value = "users", key = "#id")
public User getUserById(Long id) {
// 从数据库中获取用户信息
return userRepository.findById(id);
}
@CachePut(value = "users", key = "#user.id")
public User saveUser(User user) {
// 保存用户信息到数据库
return userRepository.save(user);
}
@CacheEvict(value = "users", key = "#id")
public void deleteUserById(Long id) {
// 从数据库中删除用户信息
userRepository.deleteById(id);
}
}
```
在上述示例中,`@Cacheable`注解用于根据用户ID查询用户信息,并将查询结果缓存起来;`@CachePut`注解用于保存用户信息到数据库,并将保存后的用户信息存入缓存;`@CacheEvict`注解用于删除用户信息,并同时从缓存中移除相应的数据。
需要注意的是,由于使用了注解缓存,所以必须保证方法的入参和返回值是可序列化的类型,以便正确地进行缓存操作。
这样,你就可以通过注解实现Spring Boot与Redis的整合了。
阅读全文
相关推荐
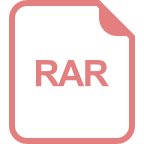
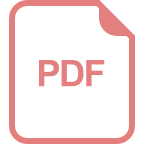


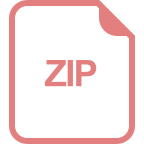
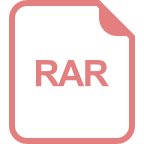
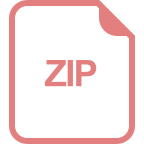
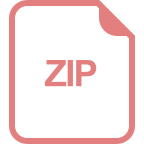
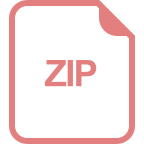
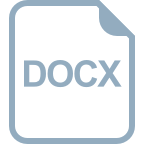
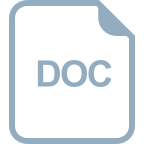



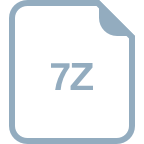
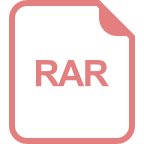