for i in len(nums): TypeError: 'int' object is not iterable
时间: 2024-09-02 16:04:17 浏览: 70
`TypeError: 'int' object is not iterable` 是因为在尝试迭代(for循环)一个整数时产生的错误。整数 `len()` 函数返回的是长度,而不是一个可迭代的对象。当你试图用 `for i in len(nums)` 的方式遍历它,实际上是期望它像列表或其他序列那样逐个元素地提供,但整数不具备这种属性。
正确的做法应该是直接使用整数值,而不是在循环里操作它。如果你想基于某个整数执行固定次数的操作,应该写成:
```python
num_iterations = len(nums)
for _ in range(num_iterations): # 使用range代替iterating over len
# 执行你的代码
```
或者如果你确实想获取列表的长度并对其进行某些操作,你应该这样写:
```python
length = len(nums)
for i in range(length): # 正确的迭代nums
# 对于 nums 中的每个索引 i 进行操作
```
相关问题
TypeError: argument of type 'int' is not iterable
This error occurs when you try to iterate over an integer value, which is not possible in Python.
For example, if you have the following code:
```
num = 10
for i in num:
print(i)
```
You will get the TypeError: argument of type 'int' is not iterable because you are trying to iterate over an integer value 'num'.
To fix this error, you need to make sure that you are iterating over an iterable object, such as a list or a tuple. For example:
```
nums = [1, 2, 3, 4, 5]
for num in nums:
print(num)
```
Here, we are iterating over the list 'nums', which is an iterable object, so we can successfully iterate over the values in the list without getting the TypeError.
for i in xrange(len(nums)):
It seems like you are trying to iterate over a sequence of numbers using a `for` loop in Python. However, the use of `xrange` suggests that you might be using Python 2.x instead of Python 3.x.
In Python 2.x, the `xrange` function generates numbers on-the-fly, which is more memory-efficient for large ranges. In Python 3.x, the equivalent function is `range`.
Here's an example of how you can use a `for` loop with `xrange` in Python 2.x to iterate over a sequence of numbers:
```python
nums = [1, 2, 3, 4, 5]
for i in xrange(len(nums)):
print(nums[i])
```
This code will iterate over the indices of the `nums` list and print each element.
阅读全文
相关推荐
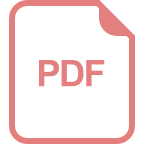
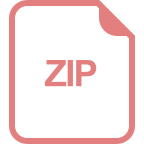
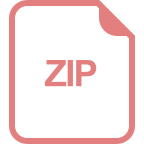














