用C++写一段在ROS2+PX4环境下让无人机绕矩形飞行的程序
时间: 2024-12-23 15:20:46 浏览: 4
在ROS2 (Robot Operating System) 和 PX4 (Pixhawk) 环境下,编写一个让无人机绕矩形飞行的C++程序需要几个关键步骤。首先,你需要熟悉ROS2的导航 stack 和px4的API,特别是对于路径规划和控制模块。
下面是一个简化的示例,展示了如何使用`rclcpp`库创建节点,并利用`geometry_msgs::Polygon`消息来表示矩形路径:
```cpp
#include <rclcpp/rclcpp.hpp>
#include <geometry_msgs/msg/polygon.hpp>
#include "px4_controllers/mavlink_interface.h"
class CircleFlightNode : public rclcpp::Node
{
public:
CircleFlightNode(const std::string & node_name)
: Node(node_name),
path_pub_(this->create_publisher<geometry_msgs::msg::Polygon>("path", 10)),
mavlink_node_(*this)
{
// 初始化矩形路径
geometry_msgs::msg::Polygon path_msg;
path_msg.points.resize(4); // 四边形需要四个点
path_msg.points[0].x = -1.0; // 左上角
path_msg.points[0].y = 1.0;
path_msg.points[1].x = -1.0; // 左下角
path_msg.points[1].y = -1.0;
path_msg.points[2].x = 1.0; // 右下角
path_msg.points[2].y = -1.0;
path_msg.points[3].x = 1.0; // 右上角
path_msg.points[3].y = 1.0;
auto timer_period = rclcpp::Duration(seconds(1));
timer_.reset(this->create_wall_timer(timer_period, [this]() -> void {
this->publish_path(path_msg);
// 在这里添加飞行控制代码,比如调用mavlink_node_的函数来发送相应指令
// 比如 PX4的move_absolute或者MissionItem
}));
}
private:
void publish_path(const geometry_msgs::msg::Polygon & path)
{
path_pub_->publish(path);
}
rclcpp::Publisher<geometry_msgs::msg::Polygon> path_pub_;
px4_mavlink_node_t mavlink_node_;
rclcpp::WallTimerSharedPtr timer_;
};
int main(int argc, char ** argv)
{
rclcpp::init(argc, argv);
try {
CircleFlightNode node("circle_flight");
rclcpp::spin_until_future_complete(node.create_future());
} catch (std::exception& e) {
RCLCPP_ERROR(get_logger(), "Error initializing node: %s", e.what());
}
rclcpp::shutdown();
return 0;
}
```
请注意,这只是一个基础框架,实际应用中还需要处理错误、状态更新、以及与PX4的实时通信,确保安全和稳定。同时,你可能需要连接到飞控硬件并适当地调整PID控制器或其他飞行控制算法来实现精确的飞行。
阅读全文
相关推荐
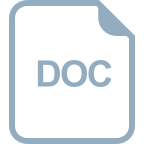
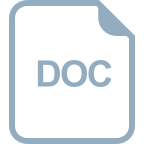
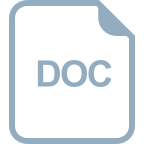
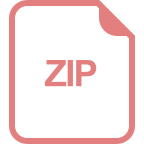
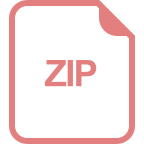
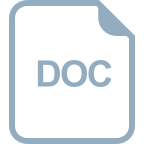
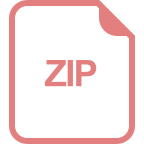
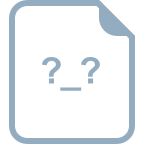
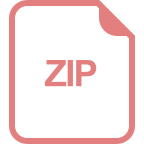
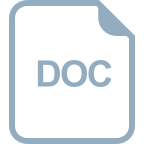





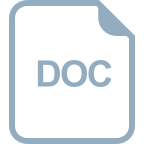
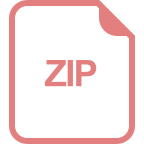
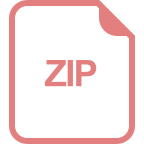