c#modbus tcp写入跟读取完整案例
时间: 2023-07-29 19:15:12 浏览: 159
当然可以!以下是一个完整的C# Modbus TCP写入和读取的案例:
```csharp
using System;
using System.Net.Sockets;
using System.Threading;
using System.Net;
namespace ModbusTCPExample
{
class Program
{
static void Main(string[] args)
{
string ipAddress = "192.168.0.1"; // Modbus设备的IP地址
int port = 502; // Modbus设备的端口号
// 创建TCP客户端
TcpClient client = new TcpClient();
client.Connect(IPAddress.Parse(ipAddress), port);
// 创建Modbus请求
ModbusTcpMaster master = new ModbusTcpMaster(client.GetStream());
// 写入数据
ushort startAddress = 0; // 起始地址
ushort[] writeData = new ushort[] { 1, 2, 3, 4 }; // 写入的数据
master.WriteMultipleRegisters(1, startAddress, writeData);
// 读取数据
ushort[] readData = master.ReadHoldingRegisters(1, startAddress, (ushort)writeData.Length);
// 打印读取到的数据
for (int i = 0; i < readData.Length; i++)
{
Console.WriteLine($"Register {startAddress + i}: {readData[i]}");
}
// 关闭TCP连接
client.Close();
Console.ReadLine();
}
}
public class ModbusTcpMaster
{
private NetworkStream stream;
public ModbusTcpMaster(NetworkStream stream)
{
this.stream = stream;
}
public void WriteMultipleRegisters(byte slaveId, ushort startAddress, ushort[] data)
{
byte[] frame = new byte[9 + data.Length * 2];
frame[0] = slaveId;
frame[1] = 0x10;
frame[2] = (byte)(startAddress >> 8);
frame[3] = (byte)startAddress;
frame[4] = (byte)(data.Length >> 8);
frame[5] = (byte)data.Length;
frame[6] = (byte)(data.Length * 2);
for (int i = 0; i < data.Length; i++)
{
frame[7 + i * 2] = (byte)(data[i] >> 8);
frame[8 + i * 2] = (byte)data[i];
}
stream.Write(frame, 0, frame.Length);
}
public ushort[] ReadHoldingRegisters(byte slaveId, ushort startAddress, ushort count)
{
byte[] frame = new byte[8];
frame[0] = slaveId;
frame[1] = 0x03;
frame[2] = (byte)(startAddress >> 8);
frame[3] = (byte)startAddress;
frame[4] = (byte)(count >> 8);
frame[5] = (byte)count;
stream.Write(frame, 0, frame.Length);
Thread.Sleep(100); // 等待数据到达
byte[] response = new byte[5 + count * 2];
stream.Read(response, 0, response.Length);
ushort[] data = new ushort[count];
for (int i = 0; i < count; i++)
{
data[i] = (ushort)((response[3 + i * 2] << 8) | response[4 + i * 2]);
}
return data;
}
}
}
```
在这个例子中,我们创建了一个ModbusTCP客户端,通过TCP连接到Modbus设备的IP地址和端口号。然后,我们使用`ModbusTcpMaster`类来执行写入和读取操作。
在写入操作中,我们需要指定从哪个寄存器开始写入,并提供要写入的数据。在读取操作中,我们需要指定从哪个寄存器开始读取,以及要读取的数量。
最后,我们打印出从设备读取到的数据。
请注意,这只是一个简单的示例,实际应用中可能需要根据具体的Modbus设备和协议进行适当的调整。
阅读全文
相关推荐
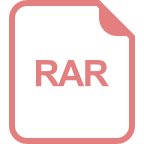
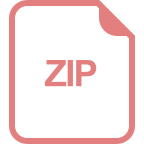
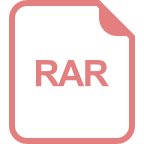
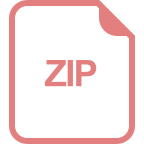
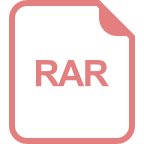
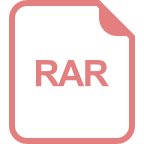







