使用C语言编程实现1. 定义单向循环链表节点类型。 2..尾插法创建单向循环链表并返回尾指针。 3.定义打印输出单向循环链表节点。 4. 在main函数中编写测试 (1)分别创建循环链表La 和Lb
时间: 2024-09-25 21:03:52 浏览: 54
在C语言中,我们可以按照以下步骤来实现单向循环链表:
1. 定义单向循环链表节点类型(Node结构体):
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. 尾插法创建单向循环链表并返回尾指针:
```c
Node* createCircularList(int arr[], int size) {
Node* head = NULL, *tail = NULL;
for (int i = 0; i < size; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = head;
if (!head)
head = tail = newNode;
else
tail->next = head, tail = newNode;
}
return head;
}
```
3. 定义打印输出单向循环链表节点:
```c
void printCircularList(Node* node) {
Node* temp = node;
do {
printf("%d ", temp->data);
temp = temp->next;
} while (temp != node);
}
```
4. 在`main`函数中编写测试示例:
```c
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
Node* La = createCircularList(arr, size);
// 打印链表La
printf("List La: ");
printCircularList(La);
// 创建另一个循环链表Lb
int arr2[] = {6, 7, 8, 9, 10};
Node* Lb = createCircularList(arr2, size);
// 打印链表Lb
printf("\nList Lb: ");
printCircularList(Lb);
free(La);
free(Lb); // 释放内存
return 0;
}
```
在这个例子中,我们首先创建了两个循环链表`La`和`Lb`,然后分别打印它们的内容。记得在程序结束时释放分配的内存。
阅读全文
相关推荐
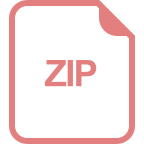
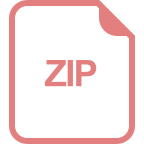
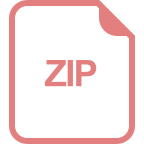









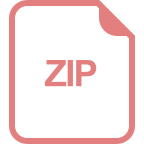
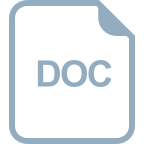
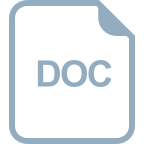
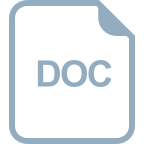


