python opencv 画矩形
时间: 2023-08-13 21:10:06 浏览: 108
你可以使用Python的OpenCV库来绘制矩形。下面是一个简单的示例代码:
```python
import cv2
# 读取图像
image = cv2.imread("image.jpg")
# 定义矩形的左上角和右下角坐标
start_point = (50, 50)
end_point = (200, 200)
# 定义矩形的颜色和线宽
color = (0, 255, 0) # 绿色
thickness = 2
# 绘制矩形
cv2.rectangle(image, start_point, end_point, color, thickness)
# 显示绘制结果
cv2.imshow("Rectangle", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,我们首先使用`cv2.imread()`函数读取了一张图像。然后,我们定义了矩形的左上角和右下角坐标,以及矩形的颜色和线宽。最后,使用`cv2.rectangle()`函数来绘制矩形,并使用`cv2.imshow()`函数显示绘制结果。
你可以将示例代码中的`image.jpg`替换为你自己的图像路径,然后运行代码即可看到绘制的矩形。
相关问题
python opencv画矩形
要在图像上绘制矩形,可以使用OpenCV的`cv2.rectangle()`函数。下面是一个简单的示例代码,展示了如何使用OpenCV绘制矩形:
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 定义矩形的起始点和结束点坐标
start_point = (100, 100)
end_point = (300, 300)
# 定义矩形的颜色和线宽
color = (0, 255, 0) # 绿色
thickness = 2
# 在图像上绘制矩形
cv2.rectangle(image, start_point, end_point, color, thickness)
# 显示绘制矩形后的图像
cv2.imshow('Image with Rectangle', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们使用`cv2.rectangle()`函数来绘制一个矩形。`start_point`和`end_point`分别指定矩形的左上角和右下角坐标。`color`指定了矩形的颜色,这里使用了RGB格式,(0, 255, 0)表示绿色。`thickness`指定了绘制矩形的线宽。
最后,通过`cv2.imshow()`函数显示绘制矩形后的图像,并通过`cv2.waitKey(0)`等待用户按下任意键关闭窗口。最后使用`cv2.destroyAllWindows()`关闭所有的窗口。
你可以根据需要调整矩形的起始点、结束点、颜色和线宽来绘制不同样式的矩形。
python opencv画矩形如何画
在Python中使用OpenCV(Open Source Computer Vision Library)绘制矩形,你可以使用`cv2.rectangle()`函数。这个函数的基本语法如下:
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 定义矩形的位置和大小 (x, y) - 矩形左上角坐标,
# width, height - 矩形的宽度和高度
position = (50, 50)
size = (100, 100)
# 定义颜色(BGR格式,这里以蓝色为例)
color = (255, 0, 0) # BGR: 蓝色通道值分别为255, 0, 0
# 绘制矩形
cv2.rectangle(image, position, (position + size, position + size), color)
# 显示结果
cv2.imshow('Image with rectangle', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,你需要替换`image.jpg`为你实际要处理的图片路径,并根据需要调整位置、尺寸和颜色。`cv2.waitKey(0)`用于暂停显示以便查看结果,`cv2.destroyAllWindows()`关闭所有窗口。
阅读全文
相关推荐
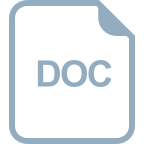
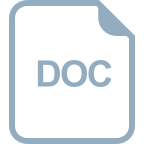
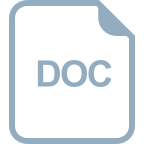










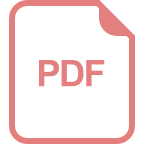


