机械臂每个关节之间的变换矩阵C语言代码
时间: 2024-12-20 10:20:28 浏览: 10
在C语言中,机械臂关节之间的变换矩阵通常涉及到旋转和平移操作,可以使用矩阵乘法来组合各个关节的影响。这里假设我们有一个基本的四元数表示的角度(q1, q2, ..., qn),对于每个关节,我们可以创建一个旋转矩阵Ri:
```c
#include <stdio.h>
#include <math.h>
// 定义一个四元数结构体
typedef struct {
double w; // 实部
double x;
double y;
double z; // 虚部
} Quaternion;
// 从角度转换到四元数
Quaternion angles_to_quaternion(double angle, double axis[3]) {
Quaternion q;
double sin_half_angle = sin(angle / 2);
q.w = cos_half_angle;
q.x = axis[0] * sin_half_angle;
q.y = axis[1] * sin_half_angle;
q.z = axis[2] * sin_half_angle;
return q;
}
// 创建旋转矩阵
Matrix4x4 rotation_matrix(Quaternion q) {
Matrix4x4 m = { {1 - 2*q.y*q.y - 2*q.z*q.z, 2*(q.x*q.y - q.w*q.z), 2*(q.x*q.z + q.w*q.y), 0},
{2*(q.x*q.y + q.w*q.z), 1 - 2*q.x*q.x - 2*q.z*q.z, 2*(q.y*q.z - q.w*q.x), 0},
{2*(q.x*q.z - q.w*q.y), 2*(q.y*q.z + q.w*q.x), 1 - 2*q.x*q.x - 2*q.y*q.y, 0},
{0, 0, 0, 1} };
return m;
}
// 组合变换矩阵
Matrix4x4 transform_chain(Matrix4x4 prev_transform, int num_joints, Quaternion* joints) {
Matrix4x4 current_transform = identity_matrix();
for (int i = 0; i < num_joints; i++) {
current_transform = multiply_matrices(current_transform, rotation_matrix(joints[i]));
}
return multiply_matrices(prev_transform, current_transform);
}
// 示例:计算单关节后的变换矩阵
Matrix4x4 single_joint_transform(double angle, double axis[3]) {
Quaternion joint_q = angles_to_quaternion(angle, axis);
return rotation_matrix(joint_q);
}
// ... 其他必要的矩阵乘法、初始化和打印函数 ...
//
阅读全文
相关推荐
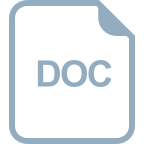
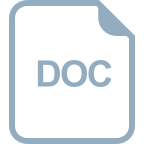
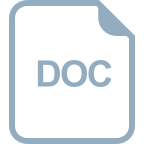
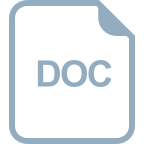
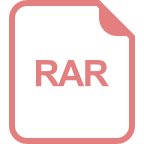
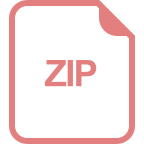
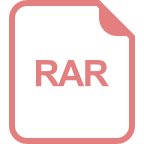
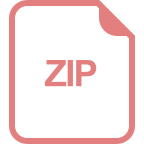
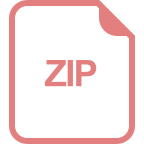
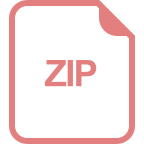
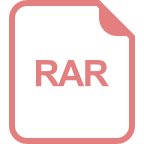
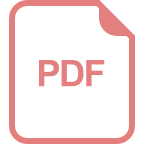
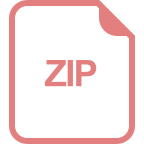
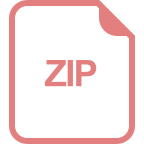
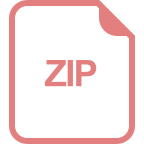
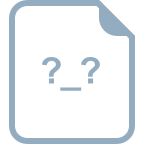