C#迭代求圆周率,e和斐波那契数列,要求给出运行结果,编写完整代码
时间: 2024-09-22 15:07:35 浏览: 66
在C#中,我们可以使用循环和数学公式来估算圆周率、计算自然常数e以及生成斐波那契数列。下面我会分别给出三种算法的简单示例代码:
1. **迭代求圆周率** (使用Leibniz公式)
```csharp
using System;
public class Program
{
public static double PIApproximation(int iterations)
{
double pi = 0.0;
for (int i = 0; i < iterations; i++)
{
pi += ((4.0 / (2 * i + 1)) - (4.0 / (2 * i + 3)));
}
return 4 * pi / (double)iterations;
}
public static void Main()
{
int iterations = 1000000; // 提供更多迭代次数以提高精度
Console.WriteLine($"Estimated value of Pi with {iterations} iterations: {PIApproximation(iterations)}");
}
}
```
运行结果会是一个近似值。
2. **计算自然常数e (欧拉数)** (使用泰勒级数)
```csharp
public class Program
{
public static double EApproximation(int terms)
{
double e = 1.0;
double factor = 1.0;
for (int i = 1; i <= terms; i++)
{
e += factor / i;
factor *= 1.0 / Math.Pow(10, i);
}
return e;
}
public static void Main()
{
int terms = 100; // 提供更多的项数以提高精度
Console.WriteLine($"Estimated value of e with {terms} terms: {EApproximation(terms):F15}");
}
}
```
运行结果会是一个近似的自然对数底数e。
3. **生成斐波那契数列**
```csharp
public class Program
{
public static IEnumerable<int> Fibonacci(int count)
{
if (count <= 0) yield break;
int a = 0, b = 1;
for (int i = 0; i < count; i++)
{
yield return a;
int temp = a;
a = b;
b = temp + b;
}
}
public static void Main()
{
int count = 10;
foreach (var fib in Fibonacci(count))
{
Console.Write($"{fib}, ");
}
Console.WriteLine();
}
}
```
运行结果会打印出指定长度的斐波那契数列。
阅读全文
相关推荐
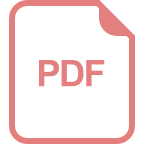
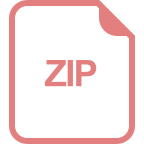
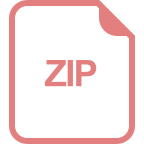

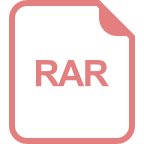
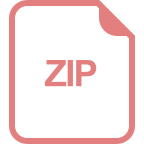
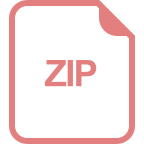
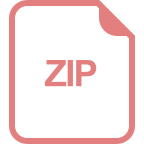
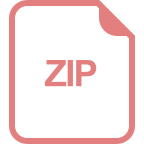
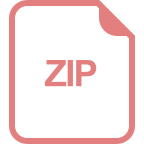
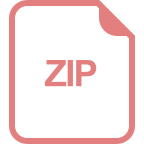
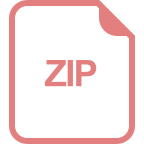
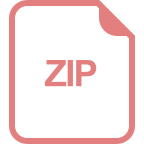
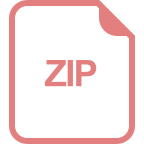
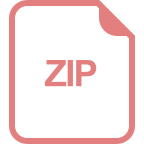
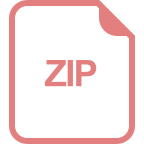
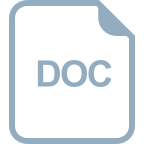


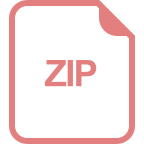