c# 实体类 多层 添加
时间: 2024-09-19 21:02:44 浏览: 4
在C#中,实体类(Entity Classes)通常用于表示数据库表中的数据模型,它们通常包含属性(字段)来存储数据,并且在多层架构(如MVC、DDD等)的应用程序中起到至关重要的作用。如果要在多层架构中添加实体类,特别是在分层设计中(例如Service Layer、Repository Layer),可以按照以下步骤操作:
1. **领域模型(Domain Model)**: 首先,你需要在业务逻辑层定义一个领域实体类,比如`Customer.cs`,它代表数据库中的"顾客"表。
```csharp
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
```
2. **数据访问层(Data Access Layer or Repository)**: 在此层,你可以创建一个针对`Customer`实体的操作接口,如`ICustomerRepository`,并提供具体实现。
```csharp
public interface ICustomerRepository
{
void Add(Customer customer);
Customer GetById(int id);
}
```
3. **服务层(Service Layer)**: 这里处理业务逻辑,会使用到`ICustomerRepository`来保存数据。例如,在`CustomerService.cs`中:
```csharp
public class CustomerService : ICustomerService
{
private readonly ICustomerRepository _repository;
public CustomerService(ICustomerRepository repository)
{
_repository = repository;
}
public void SaveCustomer(Customer customer)
{
_repository.Add(customer);
}
}
```
4. **调用服务**:在控制器(Controller)或UI层中,通过依赖注入的方式获取`CustomerService`实例并调用其`SaveCustomer`方法。
```csharp
services.AddTransient<ICustomerService, CustomerService>();
// ...
public IActionResult Create()
{
return View();
}
[HttpPost]
public IActionResult Create(CustomerFormModel model)
{
var customer = new Customer { Name = model.Name, Email = model.Email };
_customerService.SaveCustomer(customer);
// ...
}
```
在这个过程中,每个层都有明确的责任划分,使得代码更易于维护和测试。
相关推荐
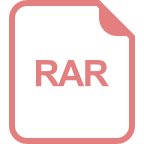
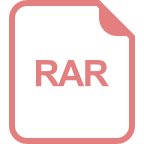
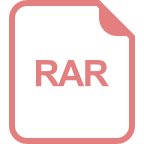














