用c语言写一段完整的代码 用插入法建立带头结点的单循环链表,实现单循环链表的初始化、求数据元素个数、插入、删除、取数据元素等操作; 把单链表以头文件的形式保存
时间: 2024-10-08 12:11:03 浏览: 35
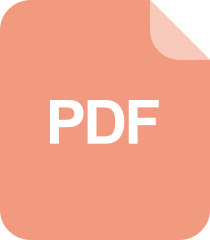
C语言单循环链表的表示与实现实例详解
在C语言中,我们首先需要创建一个结构体表示链表节点,然后编写函数实现所需的操作。这里是一个简单的单循环链表(Circular Doubly Linked List,CDLL)的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
// 初始化链表
void createCircularDLL(Node** head) {
*head = (Node*)malloc(sizeof(Node));
if (*head == NULL) {
printf("Memory allocation failed!\n");
return;
}
(*head)->data = 0; // 链表默认值
(*head)->prev = *head;
(*head)->next = *head;
}
// 求链表长度
int getLength(Node* head) {
int count = 1;
Node* temp = head;
while (temp->next != head) {
temp = temp->next;
count++;
}
return count;
}
// 插入节点
void insertAtEnd(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed!\n");
return;
}
newNode->data = value;
newNode->prev = *head;
newNode->next = (*head)->next;
(*head)->prev->next = newNode;
newNode->prev = (*head)->prev;
(*head)->next = newNode;
}
// 删除节点(假设要删除的节点已知)
void deleteNode(Node** head, int value) {
Node* current = *head;
Node* prev = NULL;
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
if (current == NULL) {
printf("Value not found in the list.\n");
return;
}
if (prev == NULL) { // 如果要删除的是头节点
*head = current->next;
free(current);
} else {
prev->next = current->next;
current->next->prev = prev;
free(current);
}
}
// 取数据元素
int getData(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return -1; // 返回无效值
}
return head->data;
}
// 测试循环链表
int main() {
Node* head = NULL;
createCircularDLL(&head);
// 执行其他操作...
// 示例:insertAtEnd(&head, 5); // 插入5
// int length = getLength(head); // 获取长度
return 0;
}
```
为了将这个功能以头文件的形式保存,你可以创建一个名为`cdll.h`的文件,包含上述函数的声明:
```c
#ifndef CDLL_H
#define CDLL_H
// 函数声明
void createCircularDLL(Node** head);
int getLength(Node* head);
void insertAtEnd(Node** head, int value);
void deleteNode(Node** head, int value);
int getData(Node* head);
#endif // CDLL_H
```
然后在另一个`.c`文件中包含这个头文件,并调用相应的函数。
阅读全文
相关推荐
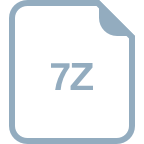
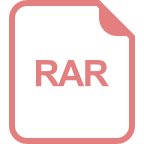











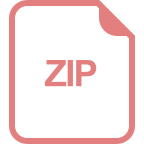
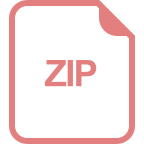