用C语言设计一个虚拟存储区和内存工作区,并使用最佳淘汰算法(OPT)、先进先出算法(FIFO)、最近最久未使用算法(LRU)计算访问命中率。
时间: 2024-09-29 07:02:24 浏览: 56
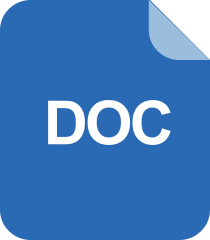
操作系统课程设计报告书-设计一个虚拟存储区和内存工作区.doc

在C语言中设计一个虚拟存储区和内存工作区涉及到一些基础的数据结构和函数,这里提供一个简化版的设计思路,我们将使用链表作为内存工作区的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个虚拟页面结构体
typedef struct Page {
int address; // 虚拟地址
char* content; // 页面内容
int accessed; // 访问标记
struct Page* next; // 指向下一个页面的指针
} Page;
// 内存工作区
typedef struct Cache {
size_t capacity; // 工作区容量
Page* head; // LRU头结点
Page* tail; // LRU尾结点
} Cache;
// 命中率计算
double calculate_hit_rate(Cache* cache, size_t page_stream_length) {
if (cache->head == NULL) return 0; // 如果为空,无访问记录
double hits = cache->capacity * cache->accessed; // 计算命中次数
double misses = page_stream_length - hits;
return 1 - misses / page_stream_length;
}
// 最佳淘汰算法模拟(无法直接实现,因需预测)
void optimal淘汰(Cache* cache, Page* page) {
printf("理想情况下,不应淘汰此页面\n");
// 实际上,这通常是通过某种预测机制实现,但C语言中不易实现
}
// 先进先出淘汰
void fifo淘汰(Cache* cache, Page* page) {
Page* temp = cache->tail;
cache->tail = cache->tail->next;
if (temp != NULL) free(temp);
temp = malloc(sizeof(Page)); // 创建新节点
temp->address = page->address;
temp->content = page->content;
temp->accessed = 1; // 设置访问标志
temp->next = cache->head;
cache->head = temp;
}
// 最近最久未使用淘汰(LRU)
void lru淘汰(Cache* cache, Page* page) {
Page* temp = cache->head;
while (temp != NULL && temp->address != page->address) {
temp = temp->next;
}
if (temp == NULL) {
printf("页面不在缓存中,无法淘汰\n");
return;
}
if (temp == cache->head) { // 如果是最前端
cache->head = temp->next;
} else {
cache->tail->next = temp->next;
}
free(temp); // 释放旧节点
temp = malloc(sizeof(Page));
temp->address = page->address;
temp->content = page->content;
temp->accessed = 1;
temp->next = cache->head;
cache->head = temp;
}
int main() {
Cache cache = {5, NULL, NULL};
// 假设我们有一个页地址流...
// 对每个页面进行访问,并更新命中率
// 示例:访问5个页面,每个页面都替换一次
for (size_t i = 0; i < 5; ++i) {
// 更新命中率
double hit_rate = calculate_hit_rate(&cache, /*page_stream_length*/);
printf("访问第 %zu 页,命中率 %.2f%%\n", i+1, hit_rate * 100);
// 使用相应淘汰算法,此处只演示LRU
Page* page = ...; // 假设得到了新访问的页面
lru淘汰(&cache, page);
}
return 0;
}
```
在这个示例中,`calculate_hit_rate`函数计算命中率,其他三个淘汰算法仅做了基本的模拟处理。实际实现中,`optimal淘汰`需要预测机制,而在C语言中并不适合。请注意,这个例子非常简略,实际应用中还需要考虑更多细节,比如页表管理、内存分配/回收等。
阅读全文
相关推荐
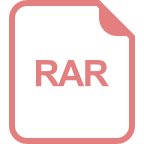
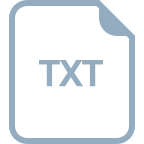










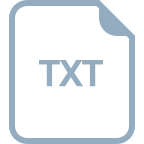
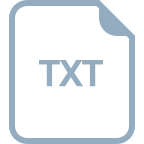
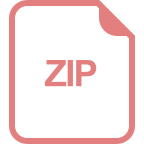
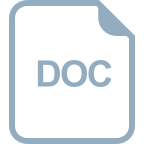
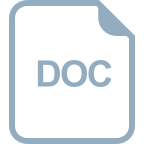
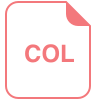
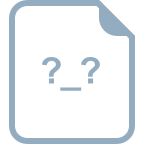