java利用shape类其派生类Rectangle实现矩形绘制,实现鼠标左键按下、拖动、松开实现自定义矩形的绘制
时间: 2024-12-22 21:26:27 浏览: 3
在Java中,你可以通过创建一个Shape接口以及它的派生类Rectangle来实现自定义矩形的绘制。首先,定义一个Shape接口,包含基本的绘制方法:
```java
public interface Shape {
void draw(Graphics g);
}
```
然后创建Rectangle类作为Shape接口的实现,添加特定于矩形的方法,如设置宽度、高度等:
```java
public class Rectangle implements Shape {
private int x;
private int y;
private int width;
private int height;
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
@Override
public void draw(Graphics g) {
g.drawRect(x, y, width, height);
}
// 添加其他可能需要的方法,如改变位置、大小等
}
```
为了实现鼠标操作(左键按下、拖动、释放),你需要监听鼠标的事件。可以使用Swing库或者Awt库中的MouseListener接口:
```java
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class CustomRectangle extends JFrame {
private Shape currentShape; // 存储当前正在绘制的形状
// ... 其他JFrame初始化和布局代码...
public CustomRectangle() {
set MouseListener(new MouseAdapter() {
@Override
public void mousePressed(MouseEvent e) {
if (currentShape != null) {
e.consume(); // 阻止默认处理(例如关闭窗口)
int newX = e.getX();
int newY = e.getY();
// 创建新的Rectangle实例并开始绘制
currentShape = new Rectangle(currentShape.getX(), currentShape.getY(), newX - currentShape.getX(), newY - currentShape.getY());
currentShape.draw(getGraphics());
} else {
// 如果没有形状,则初始化一个新的矩形
currentShape = new Rectangle(e.getX(), e.getY(), 0, 0);
}
}
//... 包含mouseDragged和mouseReleased方法,分别处理拖动和释放事件
@Override
public void mouseMoved(MouseEvent e) {
if (currentShape != null) {
currentShape.draw(getGraphics()); // 更新绘图位置
}
}
});
}
}
```
在这个例子中,当你左键点击屏幕时,会创建一个新的Rectangle,并在后续的鼠标移动和释放事件中更新矩形的位置。每次鼠标左键按下和拖动都会动态地更新矩形的大小和位置。
阅读全文
相关推荐
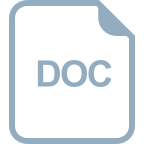
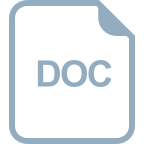
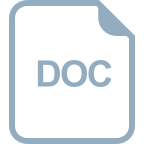

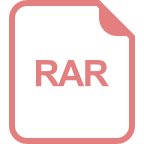
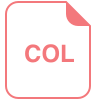
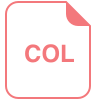
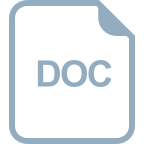
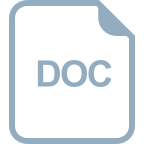
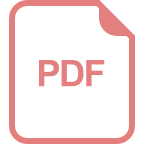
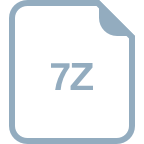
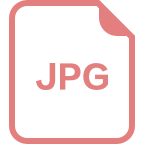
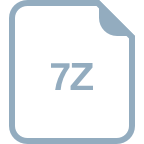
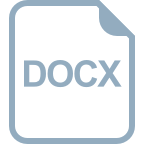