数据结构prim、kruskal、Floyd、Dijkstra算法C语言代码
时间: 2023-09-09 17:12:49 浏览: 68
对于Prim、Kruskal、Floyd和Dijkstra算法的C语言代码,我可以为您提供一些示例。请注意,以下代码仅供参考,实际应用时可能需要根据具体情况进行调整。
1. Prim算法(最小生成树):
```c
#include <stdio.h>
#include <stdbool.h>
#define INF 9999
#define V 5
int minKey(int key[], bool mstSet[]) {
int min = INF, min_index;
for (int v = 0; v < V; v++) {
if (mstSet[v] == false && key[v] < min) {
min = key[v];
min_index = v;
}
}
return min_index;
}
void printMST(int parent[], int graph[V][V]) {
printf("Edge \tWeight\n");
for (int i = 1; i < V; i++) {
printf("%d - %d \t%d \n", parent[i], i, graph[i][parent[i]]);
}
}
void primMST(int graph[V][V]) {
int parent[V];
int key[V];
bool mstSet[V];
for (int i = 0; i < V; i++) {
key[i] = INF;
mstSet[i] = false;
}
key[0] = 0;
parent[0] = -1;
for (int count = 0; count < V - 1; count++) {
int u = minKey(key, mstSet);
mstSet[u] = true;
for (int v = 0; v < V; v++) {
if (graph[u][v] && mstSet[v] == false && graph[u][v] < key[v]) {
parent[v] = u;
key[v] = graph[u][v];
}
}
}
printMST(parent, graph);
}
int main() {
int graph[V][V] = {
{0, 2, 0, 6, 0},
{2, 0, 3, 8, 5},
{0, 3, 0, 0, 7},
{6, 8, 0, 0, 9},
{0, 5, 7, 9, 0}
};
primMST(graph);
return 0;
}
```
2. Kruskal算法(最小生成树):
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define V 5
#define MAX_EDGES 10
struct Edge {
int src, dest, weight;
};
struct Graph {
int V, E;
struct Edge edges[MAX_EDGES];
};
struct Graph* createGraph(int V, int E) {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
graph->V = V;
graph->E = E;
return graph;
}
int find(int parent[], int i) {
if (parent[i] == -1)
return i;
return find(parent, parent[i]);
}
void Union(int parent[], int x, int y) {
int xset = find(parent, x);
int yset = find(parent, y);
parent[xset] = yset;
}
int compare(const void* a, const void* b) {
struct Edge* a1 = (struct Edge*)a;
struct Edge* b1 = (struct Edge*)b;
return a1->weight - b1->weight;
}
void kruskalMST(struct Graph* graph) {
int parent[V];
int i, j;
int edgeCount = 0;
qsort(graph->edges, graph->E, sizeof(graph->edges[0]), compare);
for (i = 0; i < V; i++)
parent[i] = -1;
i = 0;
while (edgeCount < V - 1 && i < graph->E) {
struct Edge nextEdge = graph->edges[i++];
int x = find(parent, nextEdge.src);
int y = find(parent, nextEdge.dest);
if (x != y) {
printf("%d - %d\t%d\n", nextEdge.src, nextEdge.dest, nextEdge.weight);
Union(parent, x, y);
edgeCount++;
}
}
}
int main() {
int V = 5;
int E = 7;
struct Graph* graph = createGraph(V, E);
graph->edges[0].src = 0;
graph->edges[0].dest = 1;
graph->edges[0].weight = 2;
graph->edges[1].src = 0;
graph->edges[1].dest = 3;
graph->edges[1].weight = 6;
// 以此类推,添加其他边的信息...
kruskalMST(graph);
return 0;
}
```
3. Floyd算法(最短路径):
```c
#include <stdio.h>
#define V 4
#define INF 99999
void printSolution(int dist[][V]);
void floydWarshall(int graph[][V]) {
int dist[V][V], i, j, k;
for (i = 0; i < V; i++) {
for (j = 0; j < V; j++) {
dist[i][j] = graph[i][j];
}
}
for (k = 0; k < V; k++) {
for (i = 0; i < V; i++) {
for (j = 0; j < V; j++) {
if (dist[i][k] + dist[k][j] < dist[i][j]) {
dist[i][j] = dist[i][k] + dist[k][j];
}
}
}
}
printSolution(dist);
}
void printSolution(int dist[][V]) {
printf("The following matrix shows the shortest distances between every pair of vertices:\n");
for (int i = 0; i < V; i++) {
for (int j = 0; j < V; j++) {
if (dist[i][j] == INF) {
printf("%7s", "INF");
} else {
printf("%7d", dist[i][j]);
}
}
printf("\n");
}
}
int main() {
int graph[V][V] = {
{0, 5, INF, 10},
{INF, 0, 3, INF},
{INF, INF, 0, 1},
{INF, INF, INF, 0}
};
floydWarshall(graph);
return 0;
}
```
4. Dijkstra算法(最短路径):
```c
#include <stdio.h>
#include <stdbool.h>
#define V 6
#define INF 9999
int minDistance(int dist[], bool sptSet[]) {
int min = INF, min_index;
for (int v = 0; v < V; v++) {
if (sptSet[v] == false && dist[v] <= min) {
min = dist[v];
min_index = v;
}
}
return min_index;
}
void printSolution(int dist[]) {
printf("Vertex \t\t Distance from Source\n");
for (int i = 0; i < V; i++) {
printf("%d \t\t %d\n", i, dist[i]);
}
}
void dijkstra(int graph[V][V], int src) {
int dist[V];
bool sptSet[V];
for (int i = 0; i < V; i++) {
dist[i] = INF;
sptSet[i] = false;
}
dist[src] = 0;
for (int count = 0; count < V - 1; count++) {
int u = minDistance(dist, sptSet);
sptSet[u] = true;
for (int v = 0; v < V; v++) {
if (!sptSet[v] && graph[u][v] && dist[u] != INF && dist[u] + graph[u][v] < dist[v]) {
dist[v] = dist[u] + graph[u][v];
}
}
}
printSolution(dist);
}
int main() {
int graph[V][V] = {
{0, 4, 0, 0, 0, 0},
{4, 0, 8, 0, 0, 0},
{0, 8, 0, 7, 0, 4},
{0, 0, 7, 0, 9, 14},
{0, 0, 0, 9, 0, 10},
{0, 0, 4, 14, 10, 0}
};
dijkstra(graph, 0);
return 0;
}
```
以上是Prim、Kruskal、Floyd和Dijkstra算法的一些示例C语言代码。希望对您有所帮助!
相关推荐
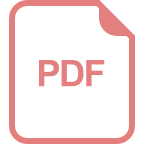
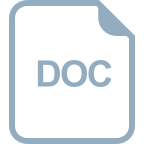
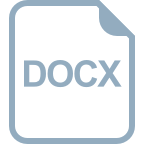














