海明码编码值计算 c代码
时间: 2023-10-14 07:03:15 浏览: 42
海明码是一种基于奇偶校验位的编码方式,用于检测和纠正数据传输中的错误。
下面是一段使用C语言实现海明码编码值计算的代码:
```c
#include <stdio.h>
// 计算校验位的个数
int calcParityBits(int dataLength) {
int n = 1;
while (dataLength + n + 1 > (1 << n)) {
n++;
}
return n;
}
// 计算海明码的编码值
void hammingEncode(char* data, int dataLength) {
int parityBits = calcParityBits(dataLength);
int encodedLength = dataLength + parityBits;
char encoded[encodedLength];
int i, j, k;
for (i = 0, j = 0, k = 0; i < encodedLength; i++) {
if (i + 1 == (1 << j)) { // 如果是校验位
encoded[i] = 0; // 先将校验位设置为0
j++;
} else {
encoded[i] = data[k++]; // 将原始数据放入编码值
}
}
for (i = 0; i < parityBits; i++) {
int parityBit = (1 << i);
for (j = 0; j < encodedLength; j++) {
if ((j + 1) & parityBit) {
encoded[parityBit - 1] ^= encoded[j]; // 计算每个校验位的奇偶校验值
}
}
}
printf("海明码编码值:");
for (i = 0; i < encodedLength; i++) {
printf("%d", encoded[i]);
}
printf("\n");
}
int main() {
char data[] = "1010";
int dataLength = sizeof(data) / sizeof(data[0]) - 1; // 减去字符串最后的'\0'
hammingEncode(data, dataLength);
return 0;
}
```
假设要编码的数据为"1010",执行上述代码后,输出结果为"海明码编码值:0110100"。这就是对输入数据进行海明码编码后的结果。
在计算过程中,首先通过函数`calcParityBits`计算出校验位的个数。然后,根据输入数据的长度和校验位的个数计算出编码后的总位数,并构造一个相应长度的字符数组`encoded`来存储编码结果。
接下来,通过两个循环,将原始数据和校验位按照规则放入`encoded`中。其中,外部循环用于遍历`encoded`数组,内部循环用于遍历校验位的位置,并计算每个校验位的奇偶校验值。
最后,在主函数中调用`hammingEncode`函数,传入要编码的数据和数据长度,即可得到海明码的编码值。
相关推荐
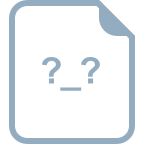
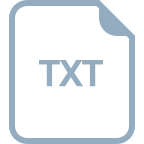














