如何用C语言编写一个链表数据结构,实现动态插入和删除功能(指定位置)?请提供一个示例,包括如何初始化链表并利用尾插法存储整数1, 2, 3, 4, 5,并展示删除和插入操作之后的链表输出。
时间: 2024-10-28 20:11:40 浏览: 5
在C语言中,我们可以使用结构体定义链表节点,并通过头指针管理整个链表。这里是一个简单的实现,包括链表的初始化、动态插入和删除:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表
Node* initializeList() {
return (Node*) malloc(sizeof(Node)); // 分配内存
}
// 插入节点到链表的指定位置
void insertAtPosition(Node** head, int position, int value) {
if (*head == NULL) { // 如果链表为空
*head = initializeList();
(*head)->data = value;
} else if (position == 0) { // 插入在头部
Node* newNode = initializeList();
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else { // 在中间或尾部插入
Node* current = *head;
for (int i = 1; i < position && current != NULL; i++) {
current = current->next;
}
if (current != NULL) {
Node* newNode = initializeList();
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
} else {
printf("Invalid position.\n");
}
}
}
// 删除链表中指定位置的节点
void deleteFromPosition(Node** head, int position) {
if (*head == NULL || position < 0) {
printf("List is empty or invalid position.\n");
return;
}
if (position == 0) { // 删除头部节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* current = *head;
for (int i = 1; i < position && current != NULL; i++) {
current = current->next;
}
if (current != NULL && current->next != NULL) { // 找到目标节点
Node* temp = current->next;
current->next = temp->next;
free(temp);
} else {
printf("Invalid position.\n");
}
}
}
// 遍历并打印链表
void printList(Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
Node* head = initializeList(); // 初始化链表
insertAtPosition(&head, 0, 1); // 插入1
insertAtPosition(&head, 1, 2);
insertAtPosition(&head, 2, 3);
insertAtPosition(&head, 3, 4);
insertAtPosition(&head, 4, 5); // 插入5
printf("Original list:\n");
printList(head);
deleteFromPosition(&head, 2); // 删除第三个节点(值为3)
printf("After deleting node at position 2:\n");
printList(head);
return 0;
}
```
在这个例子中,我们首先创建了一个空链表,然后逐个插入整数1到5。接着,我们删除了值为3的节点,最后展示了修改后的链表。
运行此程序,你会看到原始链表和删除操作后的链表输出。注意,在实际应用中,你可能需要处理更多边界情况和错误检查。
阅读全文
相关推荐
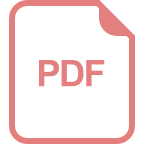
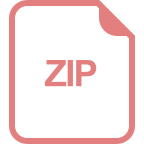
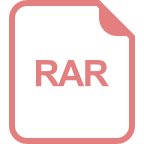

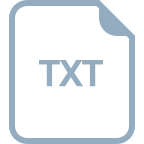
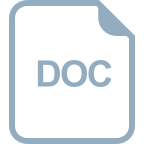
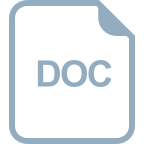
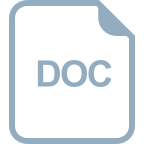
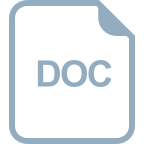
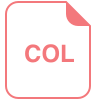
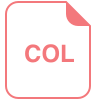







