如何用C语言编写一个链表数据结构,实现动态插入(允许指定位置)和删除功能?请提供一个示例,包括如何初始化链表存储整数1, 2, 3, 4, 5,并展示删除和插入操作之后的链表输出。
时间: 2024-10-28 20:12:02 浏览: 26
在C语言中,我们可以使用结构体定义链表节点,然后创建一个链表头节点,以及两个函数来实现动态插入和删除。以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 初始化链表,包含整数1到5
void initializeList(struct Node** head) {
*head = (struct Node*)malloc(sizeof(struct Node));
if (*head == NULL) {
printf("Memory allocation failed!\n");
return;
}
(*head)->data = 1;
struct Node* current = *head;
for (int i = 2; i <= 5; i++) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = i;
newNode->next = current->next;
current->next = newNode;
current = newNode;
}
}
// 在链表的指定位置插入元素
void insertAtPosition(struct Node** head, int position, int value) {
if (position <= 0 || *head == NULL) {
printf("Invalid insertion position.\n");
return;
}
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
struct Node* current = *head;
if (position == 1) { // 插入到头部
newNode->next = *head;
*head = newNode;
} else {
for (int i = 1; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current != NULL) {
newNode->next = current->next;
current->next = newNode;
} else {
printf("Insertion position out of range.\n");
}
}
}
// 删除链表中的某个元素
void deleteElement(struct Node** head, int value) {
if (*head == NULL) {
return;
}
struct Node* current = *head;
struct Node* prev = NULL;
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
if (current == NULL) {
printf("Value not found in the list.\n");
return;
}
if (prev == NULL) { // 如果要删除的是头节点
*head = current->next;
} else {
prev->next = current->next;
}
free(current);
}
// 打印链表
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
struct Node* head = NULL;
initializeList(&head);
printf("Initial list: ");
printList(head);
insertAtPosition(&head, 3, 6); // 插入值6在位置3
printf("After inserting at position 3: ");
printList(head);
deleteElement(&head, 3); // 删除值3
printf("After deleting value 3: ");
printList(head);
return 0;
}
```
这个程序首先初始化了一个包含整数1到5的链表,然后演示了如何插入值6到第3个位置,以及删除值3的操作,并展示了每个操作后的链表状态。
阅读全文
相关推荐
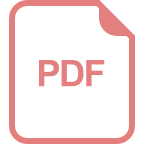
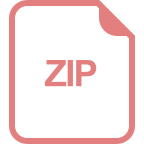
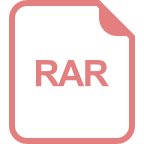

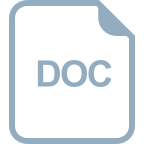
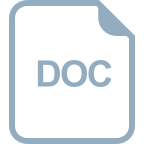
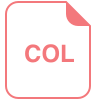











