如果使用bert大模型的框架应该如何实现
时间: 2024-12-13 13:17:38 浏览: 1
使用BERT(Bidirectional Encoder Representations from Transformers)大模型进行任务通常涉及以下几个步骤:
1. **安装依赖**:首先需要安装transformers库,它包含了许多预训练的BERT模型和相关的工具。你可以通过pip安装:
```
pip install transformers
```
2. **加载预训练模型**:从Hugging Face的仓库下载预先训练好的BERT模型。例如,对于基础的BERT模型,可以这样导入:
```python
from transformers import BertTokenizer, TFBertForSequenceClassification
model_name = "bert-base-chinese"
tokenizer = BertTokenizer.from_pretrained(model_name)
model = TFBertForSequenceClassification.from_pretrained(model_name)
```
3. **预处理输入**:对文本进行编码,包括分词和添加特殊标记。BERT需要输入两个部分:[CLS]后的句子表示和句子间的段落标记。这可以通过tokenizer.encode_plus()方法完成:
```python
def encode_text(text):
inputs = tokenizer.encode_plus(text, add_special_tokens=True, return_tensors="tf")
input_ids, token_type_ids = inputs["input_ids"], inputs["token_type_ids"]
return input_ids, token_type_ids
```
4. **模型推理**:将预处理的输入传递给模型进行分类预测:
```python
def classify_text(text, model, tokenizer):
input_ids, token_type_ids = encode_text(text)
outputs = model(inputs={
"input_ids": input_ids,
"token_type_ids": token_type_ids
})
logits = outputs.logits
predicted_class_id = tf.argmax(logits).numpy()[0]
return predicted_class_id
```
5. **训练和微调(Optional)**:如果需要,可以加载预训练模型并对其进行微调。这通常涉及到设置一个学习率衰减策略和足够的训练数据。
```python
if fine_tune:
model.trainable = True
# 开始训练过程
else:
model.trainable = False
# 使用冻结的模型进行推理
```
阅读全文
相关推荐
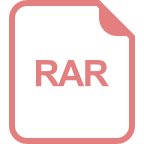
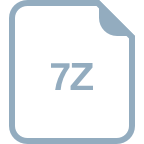
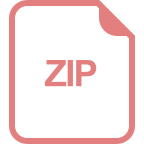
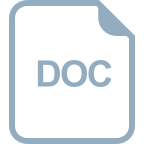
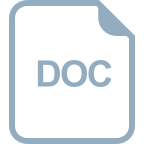
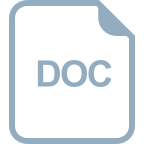
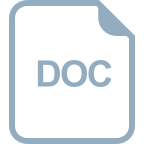


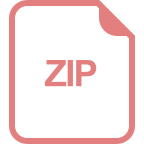
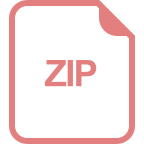
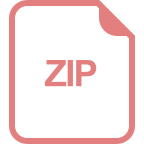
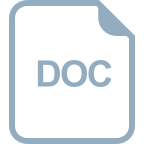
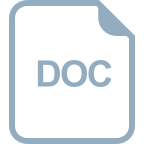
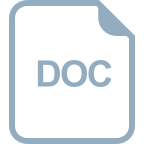


