bp神经网络鸢尾花python
时间: 2023-10-17 21:35:17 浏览: 111
你可以使用Python来实现基于BP神经网络的鸢尾花分类器。首先,你需要导入相关的库:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import OneHotEncoder
```
然后,加载鸢尾花数据集并进行预处理:
```python
# 加载数据集
data = load_iris()
X = data['data']
y = data['target']
# 将标签进行独热编码
encoder = OneHotEncoder(sparse=False)
y = encoder.fit_transform(y.reshape(-1, 1))
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,定义BP神经网络的模型:
```python
class NeuralNetwork:
def __init__(self, layers):
self.layers = layers
self.weights = [np.random.randn(layers[i], layers[i+1]) for i in range(len(layers)-1)]
self.biases = [np.random.randn(layers[i+1]) for i in range(len(layers)-1)]
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def forward_propagation(self, X):
a = X
self.layer_outputs = [a]
for i in range(len(self.layers)-2):
z = np.dot(a, self.weights[i]) + self.biases[i]
a = self.sigmoid(z)
self.layer_outputs.append(a)
z = np.dot(a, self.weights[-1]) + self.biases[-1]
a = np.exp(z) / np.sum(np.exp(z), axis=1, keepdims=True) # softmax激活函数
self.layer_outputs.append(a)
return a
def backward_propagation(self, X, y, learning_rate):
a = self.layer_outputs[-1]
delta = a - y
for i in range(len(self.layers)-2, -1, -1):
dz = delta
dw = np.dot(self.layer_outputs[i].T, dz)
db = np.sum(dz, axis=0)
delta = np.dot(dz, self.weights[i].T) * self.sigmoid_derivative(self.layer_outputs[i])
self.weights[i] -= learning_rate * dw
self.biases[i] -= learning_rate * db
def train(self, X, y, epochs, learning_rate):
for epoch in range(epochs):
output = self.forward_propagation(X)
self.backward_propagation(X, y, learning_rate)
def predict(self, X):
output = self.forward_propagation(X)
return np.argmax(output, axis=1)
```
最后,创建一个实例并进行训练和预测:
```python
# 创建一个三层的BP神经网络模型
model = NeuralNetwork([4, 10, 3])
# 训练模型
model.train(X_train, y_train, epochs=1000, learning_rate=0.1)
# 预测测试集
predictions = model.predict(X_test)
# 计算准确率
accuracy = np.mean(predictions == np.argmax(y_test, axis=1))
print("准确率:", accuracy)
```
这样就完成了使用BP神经网络进行鸢尾花分类的过程。你可以根据需要调整网络的层数和神经元数量,以及训练的迭代次数和学习率等参数来优化模型的性能。
阅读全文
相关推荐
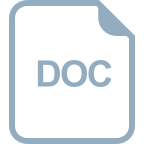
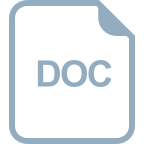
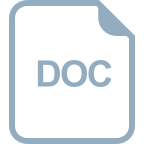

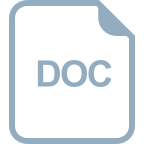
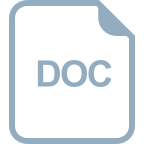
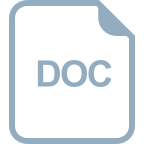
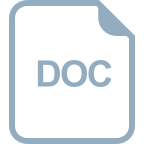
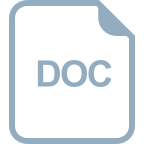





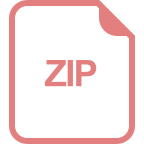

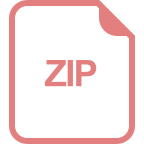