C逆位序创建单链表,实现插入,删除,输出表元素的基本操作
时间: 2024-09-14 11:05:39 浏览: 86
逆位序创建单链表意味着从尾部开始插入元素,从而构建链表。这样做的好处是不需要预先知道链表的长度。以下是创建、插入、删除和输出单链表元素的基本操作的描述:
1. 创建单链表:首先定义链表节点结构体,包含数据域和指向下一个节点的指针。然后创建一个头节点作为链表的起始点,通常头节点的数据域不存储有效数据。
2. 插入操作:创建一个新节点,然后从头节点开始遍历链表,直到找到链表的最后一个节点(即数据域为NULL的节点)。将新节点插入到这个节点之后。
3. 删除操作:遍历链表找到需要删除的节点,然后将其前一个节点的指针指向需要删除节点的下一个节点,从而移除目标节点。
4. 输出表元素:从头节点开始,遍历链表并打印每个节点的数据,直到链表结束(即下一个节点为NULL)。
以下是用C语言表示这些操作的伪代码或代码框架:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int data;
struct ListNode *next;
};
// 创建新节点
struct ListNode* createNode(int data) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 逆序创建链表
void createListReverse(struct ListNode** head, int n) {
struct ListNode* temp;
for (int i = n; i > 0; i--) {
temp = createNode(i);
// 插入到链表尾部
if (*head == NULL) {
*head = temp;
} else {
struct ListNode* last = *head;
while (last->next != NULL) {
last = last->next;
}
last->next = temp;
}
}
}
// 插入节点到链表
void insertNode(struct ListNode** head, int data) {
struct ListNode* newNode = createNode(data);
if (*head == NULL || (*head)->next == NULL) {
newNode->next = *head;
*head = newNode;
} else {
struct ListNode* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 删除链表中的节点
void deleteNode(struct ListNode** head, int key) {
struct ListNode* temp = *head, *prev = NULL;
if (temp != NULL && temp->data == key) {
*head = temp->next;
free(temp);
} else {
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) return;
prev->next = temp->next;
free(temp);
}
}
// 输出链表元素
void printList(struct ListNode* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
// 主函数示例
int main() {
struct ListNode* head = NULL;
createListReverse(&head, 5); // 创建链表 5->4->3->2->1
insertNode(&head, 6); // 插入元素 6 到链表头部
printList(head); // 输出链表 6 5 4 3 2 1
deleteNode(&head, 4); // 删除元素 4
printList(head); // 输出链表 6 5 3 2 1
return 0;
}
```
阅读全文
相关推荐
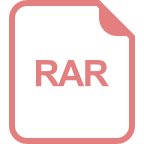
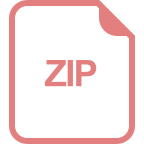
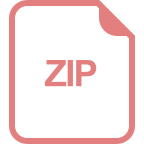


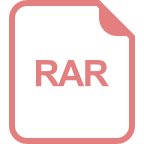
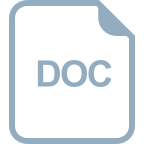
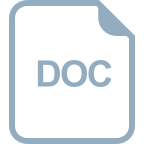
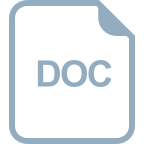
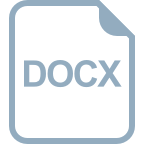
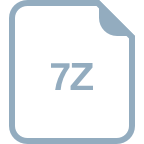
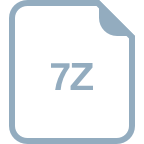
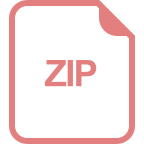
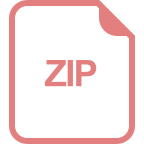