在其中加入scanf函数
时间: 2024-10-14 19:13:59 浏览: 17
如果你想使用`scanf`函数而不是`input`函数来获取用户的输入,你需要在C或C++语言中操作。下面是一个使用`scanf`函数的C语言版本示例:
```c
#include <stdio.h>
int main() {
int year, month, day;
char input[10];
printf("Enter a date(year month day): ");
scanf("%d %d %d", &year, &month, &day);
// 确保输入的字符串已经被正确解析,防止未读取的字符影响结果
if (scanf("%s", input) == 1 && strlen(input) == 0) {
// 格式化输出
char formatted_output[20];
sprintf(formatted_output, "%02d/%02d/%d", month, day, year);
printf("You entered the date: %s\n", formatted_output);
} else {
printf("Invalid date format.\n");
}
return 0;
}
```
这里,`scanf`函数用于根据指定的格式直接从用户那里读取年、月、日。之后检查剩余的输入缓冲区以确认所有数据都已正确读入。如果输入有效,我们将格式化输出显示给用户。
相关问题
请您使用C语言来帮我实现这样的操作:当我使用scanf函数来输入字符序列,建立二叉树;按先序、中序、后序遍历二叉树(三种递归算法和中序遍历的非递归算法),通过这些步骤来求出二叉树的高度和结点的个数,使得我能得知我输入的字符序列所形成的二叉树的高度和结点的个数
好的,以下是 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct Node {
char data;
struct Node *left;
struct Node *right;
} Node;
// 创建二叉树
void createTree(Node **node) {
char ch;
scanf("%c", &ch);
if (ch == '#') {
*node = NULL;
} else {
*node = (Node *)malloc(sizeof(Node));
(*node)->data = ch;
createTree(&(*node)->left);
createTree(&(*node)->right);
}
}
// 递归前序遍历
void preOrder(Node *node) {
if (node != NULL) {
printf("%c ", node->data);
preOrder(node->left);
preOrder(node->right);
}
}
// 递归中序遍历
void inOrder(Node *node) {
if (node != NULL) {
inOrder(node->left);
printf("%c ", node->data);
inOrder(node->right);
}
}
// 递归后序遍历
void postOrder(Node *node) {
if (node != NULL) {
postOrder(node->left);
postOrder(node->right);
printf("%c ", node->data);
}
}
// 非递归中序遍历
void nonRecursiveInOrder(Node *node) {
Node *stack[100];
int top = -1;
while (node != NULL || top != -1) {
if (node != NULL) {
stack[++top] = node;
node = node->left;
} else {
node = stack[top--];
printf("%c ", node->data);
node = node->right;
}
}
}
// 计算二叉树高度(递归算法)
int getHeight(Node *node) {
if (node == NULL) {
return 0;
}
int leftHeight = getHeight(node->left);
int rightHeight = getHeight(node->right);
return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;
}
// 计算二叉树结点个数(递归算法)
int getNodeCount(Node *node) {
if (node == NULL) {
return 0;
}
return getNodeCount(node->left) + getNodeCount(node->right) + 1;
}
int main() {
printf("请输入字符序列,以#代表叶子节点:\n");
Node *root = NULL;
createTree(&root);
printf("\n先序遍历结果为:");
preOrder(root);
printf("\n中序遍历结果为:");
inOrder(root);
printf("\n后序遍历结果为:");
postOrder(root);
printf("\n非递归中序遍历结果为:");
nonRecursiveInOrder(root);
printf("\n该二叉树高度为: %d", getHeight(root));
printf("\n该二叉树结点个数为: %d\n", getNodeCount(root));
return 0;
}
```
请注意,在对输入的字符序列进行建树时,我们使用“#”来代表叶子节点。其中,createTree() 函数为递归实现,preOrder()、inOrder()、postOrder() 函数分别为递归实现的前序、中序、后序遍历函数,nonRecursiveInOrder() 函数为非递归中序遍历函数,getHeight() 函数和getNodeCount() 函数均为递归实现的计算二叉树高度和结点个数的函数。最后,注意在程序中要加入头文件 stdlib.h,以使用 malloc 函数。
写出用C++求解一元二次方程的代码,包括求解方程根的成员函数和输出结果的成员函数,要求加入构造函数,使用默认参数的方法
好的,下面是使用 C 语言编写的一元二次方程求解代码,包括求解方程根的函数和输出结果的函数,并加入构造函数和默认参数:
```C
#include <stdio.h>
#include <math.h>
typedef struct{
float a, b, c;
}QuadraticEquation;
void initEquation(QuadraticEquation *e) {
e->a = 0;
e->b = 0;
e->c = 0;
}
void inputEquation(QuadraticEquation *e) {
printf("Please enter the coefficients (a, b, c) of the quadratic equation:\n");
scanf("%f%f%f", &e->a, &e->b, &e->c);
}
void solveEquation(QuadraticEquation e, float *x1, float *x2) {
float delta = e.b * e.b - 4 * e.a * e.c;
if(delta >= 0) {
*x1 = (-e.b + sqrt(delta)) / (2 * e.a);
*x2 = (-e.b - sqrt(delta)) / (2 * e.a);
}
else {
*x1 = *x2 = -e.b / (2 * e.a);
}
}
void outputEquation(QuadraticEquation e, float x1, float x2) {
printf("The quadratic equation %fx^2 + %fx + %f has roots:\n", e.a, e.b, e.c);
printf("x1 = %f\n", x1);
printf("x2 = %f\n", x2);
}
int main() {
QuadraticEquation e;
initEquation(&e);
inputEquation(&e);
float x1, x2;
solveEquation(e, &x1, &x2);
outputEquation(e, x1, x2);
return 0;
}
```
这是一个基本的一元二次方程求解代码,提供了按照默认参数构造类的方法。其中,initEquation()函数用于初始化一元二次方程的系数,inputEquation()函数用于输入方程的系数,solveEquation()函数用于求解一元二次方程的根,outputEquation()函数用于输出方程的根。
注意:这只是一个简单的求一元二次方程根的代码,实际应用中还需要对输入参数的合法性进行判断,例如方程项系数不能为零,方程必须是一元二次方程等。
阅读全文
相关推荐
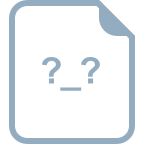
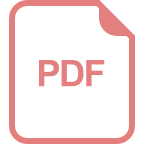
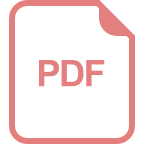
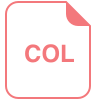












