内容1: 模拟一个“情感分析”程序,判断输入的句子(字符串)是“积极”的还是“消极”的。提示可以使用简单的关键字匹配(strstr)来判断情感色彩。 用c语言完成以上任务
时间: 2024-09-25 08:16:07 浏览: 51
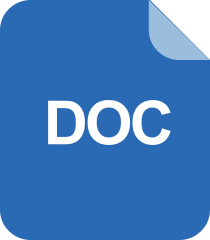
CLOB 字段类型报错 ORA-01704: 文字字符串过长的解决

在C语言中,我们可以创建一个简单的情感分析程序,通过关键字匹配来识别积极和消极的词汇。以下是一个简化的示例,使用`strstr`函数搜索关键词,并根据结果做出判断:
```c
#include <stdio.h>
#include <string.h>
#define POSITIVE_WORDS {"喜欢", "好", "棒", "高兴"} // 积极词汇列表
#define NEGATIVE_WORDS {"讨厌", "坏", "糟", "难过"} // 消极词汇列表
int is_positive(char *sentence) {
const char **positive_list = POSITIVE_WORDS;
for (const char *word : positive_list) {
if (strstr(sentence, word)) { // 查找包含的单词
return 1; // 如果找到积极词,返回1
}
}
return 0; // 否则返回0
}
int is_negative(char *sentence) {
const char **negative_list = NEGATIVE_WORDS;
for (const char *word : negative_list) {
if (strstr(sentence, word)) { // 查找包含的单词
return 1; // 如果找到消极词,返回1
}
}
return 0; // 否则返回0
}
int main() {
char sentence[100];
printf("请输入一句话:");
fgets(sentence, sizeof(sentence), stdin); // 读取用户输入
sentence[strcspn(sentence, "\n")] = '\0'; // 去除行尾的换行符
int sentiment = is_positive(sentence) ? 1 : is_negative(sentence); // 判断情感
if (sentiment == 1) {
printf("这句话是积极的。\n");
} else {
printf("这句话是消极的。\n");
}
return 0;
}
```
这个程序只是一个非常基础的示例,实际的情感分析会更复杂,需要考虑词语的上下文和语法结构。
阅读全文
相关推荐
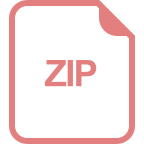
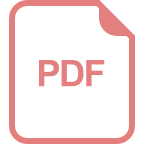















