c语言实现.在有序列表类中实现线性表抽象数据类型中的所有方法,并且实现slice方法。该方法接收start和stop两个参数,并且返回一个从start位置开始,到stop位置结束的新列表(不包含stop位置上的元素)。主程序定义实例,并对类中所有方法进行简单测试,保证算法正确性。
时间: 2024-09-17 21:08:48 浏览: 51
在C语言中,我们可以创建一个名为`OrderedList`的结构体来实现线性表抽象数据类型的动态数组版本。这个类将包括插入、删除、查找以及`slice`方法。这里是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct {
Node* head;
int size;
} OrderedList;
// 插入方法,添加新元素到链表末尾
void insert(OrderedList* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node* temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
list->size++;
}
// 查找方法,按顺序搜索给定值
int search(OrderedList* list, int value) {
Node* temp = list->head;
while (temp != NULL) {
if (temp->data == value) {
return 1; // 找到了,返回1
}
temp = temp->next;
}
return 0; // 没找到,返回0
}
// 删除方法,从链表中移除指定值
void delete(OrderedList* list, int value) {
if (list->head == NULL) {
return;
}
if (list->head->data == value) {
Node* temp = list->head;
list->head = list->head->next;
free(temp);
list->size--;
return;
}
Node* prev = list->head;
Node* current = list->head->next;
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
if (current != NULL) {
prev->next = current->next;
free(current);
list->size--;
}
}
// Slice方法,返回从start到stop的新列表
OrderedList* slice(OrderedList* list, int start, int stop) {
OrderedList* slicedList = (OrderedList*)malloc(sizeof(OrderedList));
slicedList->head = NULL;
slicedList->size = 0;
if (start < 0 || stop > list->size) {
printf("Invalid slice range.\n");
return NULL;
}
if (start == 0 && stop == list->size) {
slicedList->head = list->head;
} else {
Node* temp = list->head;
for (int i = 0; i < start; i++) {
temp = temp->next;
}
slicedList->head = temp;
temp = temp->next;
for (int i = 0; i < stop - start - 1; i++) {
slicedList->size++;
slicedList->head->next = temp;
temp = temp->next;
}
slicedList->head->next = NULL;
}
return slicedList;
}
int main() {
OrderedList list;
list.head = NULL;
list.size = 0;
// 初始化并测试操作
insert(&list, 5);
insert(&list, 2);
insert(&list, 9);
insert(&list, 1);
printf("List: ");
Node* temp = list.head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
int found = search(&list, 7); // 应该返回0
if (found) {
printf("Found 7 in the list.\n");
} else {
printf("Did not find 7 in the list.\n");
}
delete(&list, 2); // 删除2
slicedList = slice(&list, 1, 4); // 创建[1, 5, 9]的新列表
printf("Sliced List: ");
temp = slicedList->head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
return 0;
}
```
在这个示例中,我们首先初始化了一个有序列表,并测试了插入、查找和删除操作。然后,我们调用`slice`方法生成一个新的子列表,并打印结果。
阅读全文
相关推荐
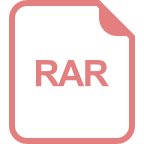
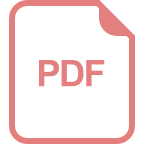
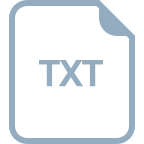
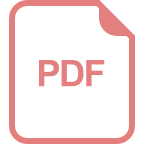
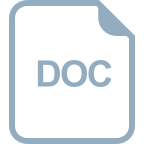
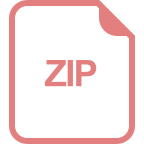
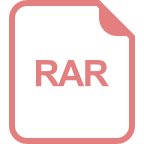
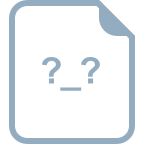
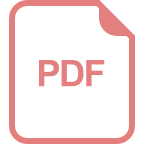
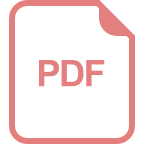
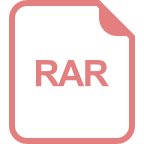
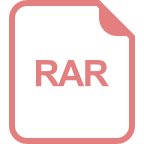
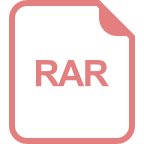
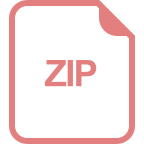