c++ curl 发送
时间: 2024-08-04 14:01:18 浏览: 121
在 C++ 中,使用 libcurl 库发送 HTTP 请求是一种常见的网络通信操作。libcurl 是一个功能强大的库,支持多种协议如 HTTP、HTTPS、FTP等。以下是一个简单的例子,展示如何使用 libcurl 发送 GET 或 POST 请求:
```cpp
#include <curl/curl.h>
size_t write_callback(char *data, size_t size, size_t nmemb, std::string* userp) {
(*userp).append(data, size * nmemb);
return size * nmemb;
}
void send_http_request(const char* url, const char* request_type, const char* data = nullptr) {
CURL* curl = curl_easy_init();
if (curl) {
std::string response;
curl_easy_setopt(curl, CURLOPT_URL, url);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback); // 设置回调函数接收响应
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
if (request_type == "POST") {
curl_easy_setopt(curl, CURLOPT_POST, 1);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
// 如果需要设置请求头,可以添加下面这行
// curl_easy_setopt(curl, CURLOPT_HTTPHEADER, ...);
}
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
} else {
printf("Response: %s\n", response.c_str());
}
curl_easy_cleanup(curl);
}
}
```
在这个示例里,`send_http_request` 函数接受 URL、请求类型(GET or POST)、以及可选的数据。对于 POST 请求,还需要设置 `CURLOPT_POSTFIELDS` 来传递数据。
使用这个基础框架,你可以根据实际需求调整参数和请求内容。例如,如果你想发送 JSON 数据,可以先将其编码成字符串作为 POST 数据。
阅读全文
相关推荐
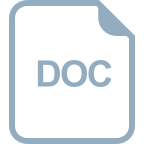
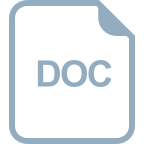
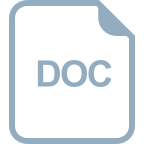

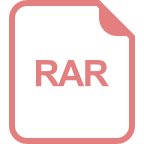













