在catch.cpp测试框架中,存在3个测试文件test_mutex.cpp、test_sem.cpp、test_task.cpp,如何把test_main.cpp文件中的函数或者变量,给到这些测试中使用?
时间: 2024-11-14 18:24:30 浏览: 3
在`catch.cpp`测试框架中,通常使用模块化的设计思想,每个单独的测试文件如`test_mutex.cpp`、`test_sem.cpp`和`test_task.cpp`关注特定的功能或模块。如果要在`test_main.cpp`中定义的函数或变量需要供这些测试文件使用,可以采用以下几种方式:
1. 公开全局变量:在`test_main.cpp`中声明并初始化那些需要共享的变量,然后在`CMakeLists.txt`中设置为公共可见。其他测试文件可以直接引用这些全局变量。
```cpp
// test_main.cpp
extern std::mutex globalMutex;
// 然后在test_mutex.cpp中使用
void someFunction() {
std::lock_guard<std::mutex> lock(globalMutex);
// ...
}
```
2. 函数原型和实现分离:在`test_main.cpp`中提供函数的声明(无实现),然后在相应的测试文件里包含该头文件并实现它。
```cpp
// test_main.cpp
void initGlobalFunctions();
// test_mutex.cpp
#include "test_main.cpp" // 包含函数声明
int main() {
initGlobalFunctions();
// ...
}
// 在test_main.cpp中实现函数
void initGlobalFunctions() {
// 初始化函数...
}
```
3. 使用依赖注入:在`test_main.cpp`中创建一个专门的类或结构体,并提供构造函数或成员函数来设置共享资源。测试文件通过实例化这个类来访问这些功能。
```cpp
// test_main.cpp
class TestContext {
public:
explicit TestContext(std::mutex& mutex) : myMutex(mutex) {}
void someFunction() { ... }
private:
std::mutex& myMutex;
};
// test_mutex.cpp
TestContext context(globalMutex);
context.someFunction();
```
无论哪种方法,都建议避免全局状态过多,保持良好的封装性和单一职责原则。同时记得在每个测试运行结束后清理资源,以防止潜在的内存泄漏。
阅读全文
相关推荐
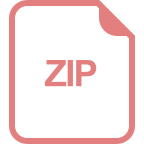
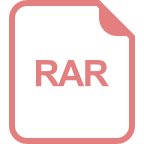
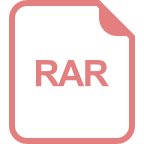















