java中将文件压缩
时间: 2024-10-11 11:00:22 浏览: 23
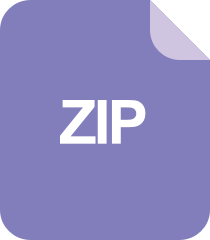
JAVA文件压缩与解压缩实践(源代码+论文)
在Java中,可以使用内置的`java.util.zip`包来实现文件的压缩。以下是几种常见的文件压缩方法:
1. **使用`ZipOutputStream`进行GZIP压缩**:
GZIPOutputStream允许你将数据压缩并写入到一个流中,可以用来压缩整个目录或单个文件:
```java
try (FileInputStream fis = new FileInputStream(file);
ZipOutputStream zos = new GZIPOutputStream(new FileOutputStream(compressedFile))) {
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) != -1) {
zos.write(buffer, 0, length);
}
} catch (IOException e) {
// handle exception
}
```
2. **使用`ZipFile`进行zip压缩**:
可以使用`ZipEntry`和`ZipOutputStream`来创建一个新的`.zip`文件:
```java
try (FileOutputStream fos = new FileOutputStream(zipFile);
ZipOutputStream zos = new ZipOutputStream(fos)) {
ZipEntry entry = new ZipEntry(originalFileName);
zos.putNextEntry(entry);
Files.copy(file.toPath(), entry.getName(), StandardCopyOption.REPLACE_EXISTING);
zos.closeEntry();
} catch (IOException e) {
// handle exception
}
```
3. **使用第三方库如Apache Commons Compress**:
如果对标准API不够满意,可以考虑使用像Apache Commons Compress这样的库,它提供了更丰富的功能。
阅读全文
相关推荐
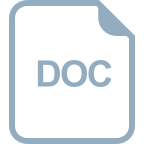
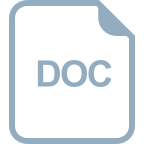
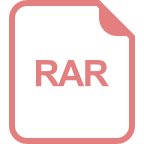
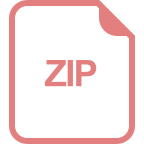
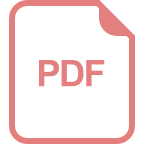
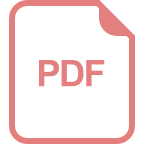
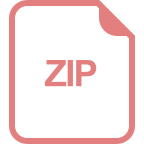
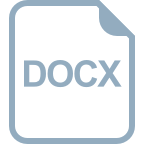
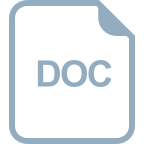
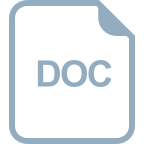
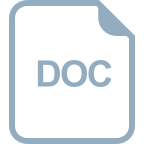
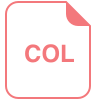



