java压缩文件夹为rar
时间: 2023-10-25 21:03:21 浏览: 127
要在Java中将文件夹压缩为RAR格式,需要使用第三方库,如 Apache Commons Compress 或 TrueZip。下面是使用 Apache Commons Compress 实现的简单示例:
1. 首先,确保你已经添加了 Apache Commons Compress 的依赖项到你的项目中。
2. 创建一个压缩文件的方法,将文件夹路径作为参数传入:
```java
import org.apache.commons.compress.archivers.ArchiveEntry;
import org.apache.commons.compress.archivers.ArchiveOutputStream;
import org.apache.commons.compress.archivers.ArchiveStreamFactory;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.zip.ZipEntry;
public class RARCompressor {
public static void compressFolderToRAR(String folderPath) {
try {
String rarPath = folderPath + ".rar";
FileOutputStream fos = new FileOutputStream(rarPath);
ArchiveOutputStream aos = new ArchiveStreamFactory().createArchiveOutputStream(ArchiveStreamFactory.RAR, fos);
Files.walk(Paths.get(folderPath))
.filter(Files::isRegularFile)
.forEach(path -> {
String entryName = folderPath + File.separator + path.getFileName();
try {
ArchiveEntry entry = aos.createArchiveEntry(new File(entryName), entryName);
aos.putArchiveEntry(entry);
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(path.toFile()));
byte[] buffer = new byte[1024];
int readBytes;
while ((readBytes = bis.read(buffer)) > 0) {
aos.write(buffer, 0, readBytes);
}
aos.closeArchiveEntry();
bis.close();
} catch (Exception e) {
e.printStackTrace();
}
});
aos.finish();
aos.close();
System.out.println("文件夹已成功压缩为RAR格式:" + rarPath);
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String folderPath = "your_folder_path";
compressFolderToRAR(folderPath);
}
}
```
3. 在 `compressFolderToRAR` 方法中,我们首先创建一个 `FileOutputStream` 对象和 `ArchiveOutputStream` 对象,用于将文件夹压缩为RAR格式。
4. 使用 `Files.walk` 遍历文件夹下的所有文件,并对每个文件进行以下操作:
a. 创建一个与文件相对应的 `ArchiveEntry` 对象。
b. 将该 `ArchiveEntry` 添加到 `ArchiveOutputStream` 中。
c. 创建一个 `BufferedInputStream` 对象读取文件内容,并使用 `ArchiveOutputStream` 将内容写入压缩文件。
d. 关闭文件输入流和 `ArchiveEntry`。
5. 在压缩完成后,关闭 `ArchiveOutputStream`,并打印出成功压缩的RAR文件路径。
请记住,这只是一个简单的示例,实际应用中可能需要处理更多的异常情况和配置选项。
阅读全文
相关推荐
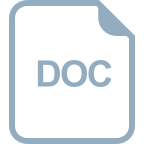
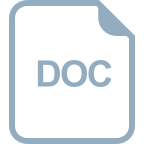
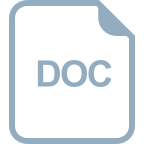

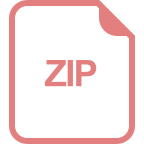
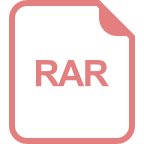
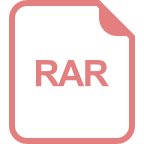
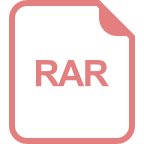
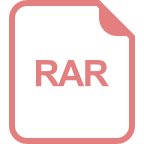
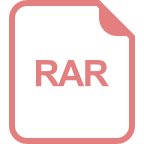
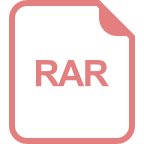
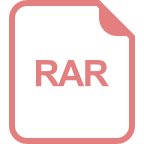
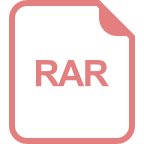
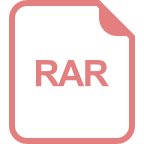
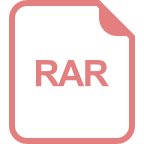
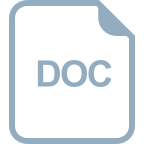


