定义一个包含图书信息(书号、书名、价格)的链表,读入相应的图书数据来完成图书信息表的创建,然后查找价格最高的图书,输出相应图书的信
时间: 2024-10-11 08:17:04 浏览: 31
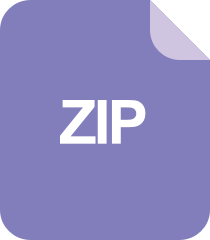
基于线性表的图书管理系统 源代码 顺序表&链表
首先,我们可以定义一个简单的链表结构,用于存储图书的信息,例如:
```python
class BookNode:
def __init__(self, isbn, title, price):
self.isbn = isbn
self.title = title
self.price = price
self.next = None
class BookList:
def __init__(self):
self.head = None
def insert_book(self, isbn, title, price):
new_node = BookNode(isbn, title, price)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def find_most_expensive_book(self):
if not self.head:
return "书籍列表为空"
max_price = self.head.price
most_expensive = self.head
current = self.head.next
while current:
if current.price > max_price:
max_price = current.price
most_expensive = current
current = current.next
return f"价格最高的图书是:{most_expensive.title},价格为 {most_expensive.price}"
```
在这个定义中,`BookNode`代表单个节点,包含书号、书名和价格;`BookList`包含了链表的基本操作,如插入新书和找到最贵的书。
要完成图书信息表的创建并查找价格最高图书,可以按照以下步骤:
1. 创建一个`BookList`实例。
2. 使用循环或从文件读取数据,根据需要调用`insert_book`方法插入新书。
3. 调用`find_most_expensive_book`方法获取价格最高的图书信息。
如果你有具体的图书数据想要示例化,我可以展示如何操作。
阅读全文
相关推荐
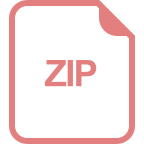
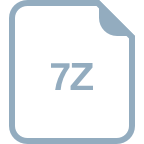












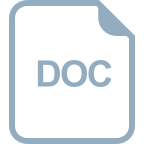

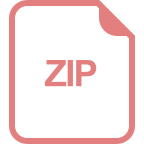