定义一个包含图书信息(书号、书名、价格)的链表,读入相应的图书数据来完成图书信息表的创建。然后,统计图书表中的个数,同时逐行输出每本图书的信息。 完整代码
时间: 2023-12-24 14:02:18 浏览: 45
我会尽力回答您的问题,以下是一个 C++ 实现的包含图书信息的链表:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Book {
string book_id;
string book_name;
double book_price;
Book* next;
};
Book* create_book_list(int n) {
Book* head = new Book;
head->next = nullptr;
Book* p = head;
for (int i = 1; i <= n; ++i) {
Book* q = new Book;
cout << "Please enter book id, book name, book price for book " << i << ":" << endl;
cin >> q->book_id >> q->book_name >> q->book_price;
q->next = nullptr;
p->next = q;
p = q;
}
return head;
}
void print_book_list(Book* head) {
Book* p = head->next;
while (p) {
cout << p->book_id << " " << p->book_name << " " << p->book_price << endl;
p = p->next;
}
}
int count_book_list(Book* head) {
int count = 0;
Book* p = head->next;
while (p) {
++count;
p = p->next;
}
return count;
}
int main() {
cout << "Please enter the number of books:" << endl;
int n;
cin >> n;
Book* head = create_book_list(n);
int book_count = count_book_list(head);
cout << "There are " << book_count << " books in the book list." << endl;
cout << "The book list is:" << endl;
print_book_list(head);
return 0;
}
```
以上代码可以创建一个包含图书信息的链表,并输出每本图书的信息。
相关推荐
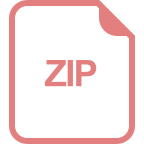
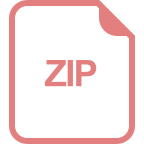
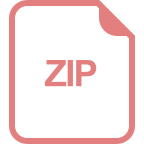








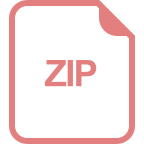
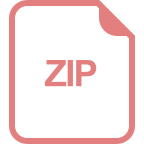
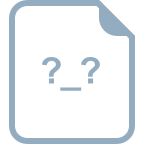
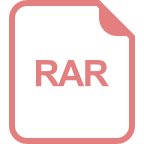
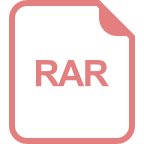