实现线性表的顺序存储结构。实现线性表在顺序存储结构下的插入和删除操作。并用该存储结构实现集合A和集合B的并集和交集操作,要求最终结果存储于集合A当中。
时间: 2024-09-22 22:07:26 浏览: 17
在计算机科学中,线性表的顺序存储结构通常是指数组这种数据结构。它通过连续的一段内存空间来存储元素,每个元素都有一个确定的索引位置。以下是基本的顺序存储结构以及插入、删除和并集、交集操作的实现:
1. **实现顺序存储结构:**
- 创建一个固定大小的数组 `list` 和两个指针 `head` 和 `tail` 分别指向列表的起始和结束位置。
```python
class SequentialList:
def __init__(self, size):
self.size = size
self.list = [None] * size
self.head = 0
self.tail = 0
```
2. **插入操作(Insertion):**
- 如果还有空余的位置,将新元素插入到 `tail+1` 的位置,然后更新 `tail` 指针。
```python
def insert(self, value):
if self.tail < self.size - 1:
self.list[self.tail + 1] = value
self.tail += 1
else:
print("List is full and cannot insert.")
```
3. **删除操作(Deletion):**
- 如果要删除的是第一个元素(即 `head` 指向),则将 `head` 移动到下一个元素;如果要删除的是最后一个元素,则仅当 `head` 不等于 `tail` 时才进行。
```python
def remove(self, value):
while self.head < self.tail and self.list[self.head] != value:
self.head += 1
if self.head == self.tail:
print("Value not found in the list.")
else:
self.head += 1
```
4. **并集操作(Union):**遍历两个列表,将非重复的元素添加到集合A中。
```python
def union(self, other_list):
for value in other_list.list:
if value not in self.list:
self.insert(value)
```
5. **交集操作(Intersection):**同样遍历两个列表,找到同时出现在两个列表中的元素,并只将它们添加到集合A中。
```python
def intersection(self, other_list):
intersection_set = set()
for value in self.list:
if value in other_list.list:
intersection_set.add(value)
# 将集合转换回顺序列表
for value in intersection_set:
self.insert(value)
```
现在,你可以创建两个 `SequentialList` 对象 A 和 B,并分别执行上述操作。
相关推荐
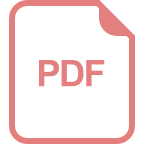
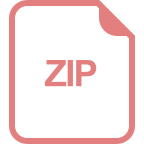
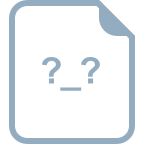














