RedisTemplate 锁代码
时间: 2024-04-24 17:26:04 浏览: 110
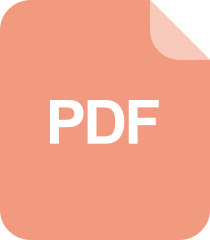
Redis Template实现分布式锁的实例代码

当使用 Spring Data Redis 框架的 RedisTemplate 来实现 Redis 锁时,可以参考以下示例代码:
```java
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import java.util.concurrent.TimeUnit;
public class RedisLock {
private RedisTemplate<String, String> redisTemplate;
private String lockKey;
private String lockValue;
private long expireTime;
public RedisLock(RedisTemplate<String, String> redisTemplate, String lockKey, long expireTime) {
this.redisTemplate = redisTemplate;
this.lockKey = lockKey;
this.expireTime = expireTime;
this.lockValue = UUID.randomUUID().toString(); // 生成唯一的锁值
}
public boolean tryLock() {
ValueOperations<String, String> valueOperations = redisTemplate.opsForValue();
Boolean success = valueOperations.setIfAbsent(lockKey, lockValue, expireTime, TimeUnit.MILLISECONDS);
return success != null && success;
}
public void unlock() {
if (lockValue.equals(redisTemplate.opsForValue().get(lockKey))) {
redisTemplate.delete(lockKey);
}
}
}
```
使用示例:
```java
public class ExampleService {
private RedisTemplate<String, String> redisTemplate;
public ExampleService(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void doSomethingWithLock() {
RedisLock lock = new RedisLock(redisTemplate, "lock-key", 5000); // 锁定 5 秒钟
try {
if (lock.tryLock()) {
// 获取到锁,执行需要加锁的操作
// ...
} else {
// 未获取到锁,处理获取锁失败的逻辑
// ...
}
} finally {
lock.unlock(); // 释放锁
}
}
}
```
请注意,以上代码仅为示例,实际使用时可能需要根据具体业务场景进行调整。同时,为了保证 RedisTemplate 的正确配置和初始化,您需要在 Spring 配置文件中配置 RedisTemplate 的 Bean。
希望这个示例对您有所帮助!如果您有更多问题,请随时提问。
阅读全文
相关推荐
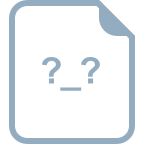
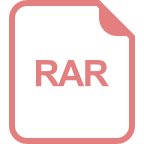






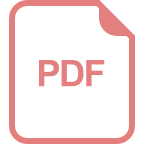
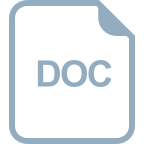
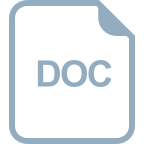




