java代码中操作远程服务器,将本地文件上传到HDFS上的示例代码
时间: 2024-09-27 17:04:32 浏览: 41
在Java中,通常我们会使用Hadoop的`FileSystem` API 来操作HDFS,包括从本地上传文件。以下是一个简单的示例,假设已经配置了Hadoop环境,并导入了必要的Hadoop库:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class HdfsUploader {
public static void main(String[] args) throws Exception {
// 创建Hadoop配置
Configuration conf = new Configuration();
// 设置HDFS地址,这里以本地模式为例,如果是生产环境需要替换为实际的URL
String hdfsUrl = "hdfs://localhost:9000";
FileSystem fs = FileSystem.get(conf);
// 指定要上传的本地文件路径和目标HDFS路径
String localFilePath = "/path/to/local/file.txt";
Path hdfsTargetPath = new Path(hdfsUrl + "/target/path/file.txt");
// 检查文件是否存在,如果不存在则创建目录
if (!fs.exists(hdfsTargetPath.getParent())) {
fs.mkdirs(hdfsTargetPath.getParent());
}
// 使用FSDataInputStream读取本地文件,然后使用FSDataOutputStream写入HDFS
FileInputStream in = new FileInputStream(localFilePath);
FSDataOutputStream out = fs.create(hdfsTargetPath);
byte[] buffer = new byte[4096];
int length;
while ((length = in.read(buffer)) != -1) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("File uploaded successfully to " + hdfsTargetPath.toString());
}
}
```
阅读全文
相关推荐
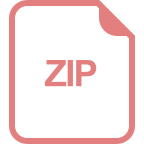
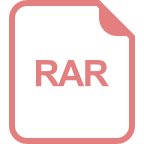
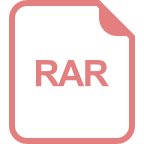

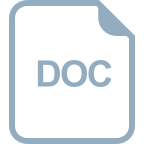
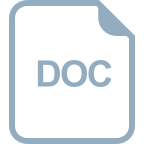
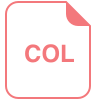
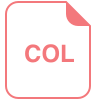
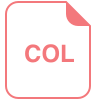
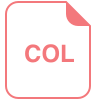
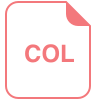
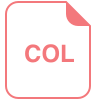
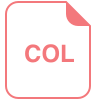
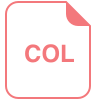
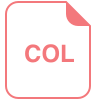
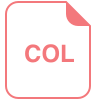
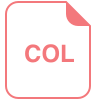
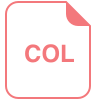
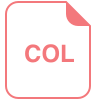