有两个矩阵a和b,均为2行3列。求两个矩阵之和。重载运算符“+”,使之能用于矩阵相加。如: c=a+b。
时间: 2024-12-15 15:26:16 浏览: 11
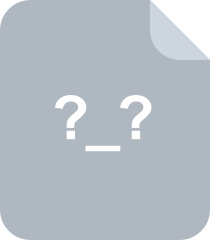
有两个矩阵a和b,均为2行3列。
在C++中,如果你想要定义一个类来表示矩阵并且能够重载加法运算符`+`来实现矩阵的相加,你可以这样做:
首先,定义一个二维数组类Matrix来存储矩阵的数据,并提供必要的属性(例如行数和列数):
```cpp
#include <vector>
class Matrix {
private:
int rows;
int cols;
std::vector<std::vector<double>> data;
public:
Matrix(int r, int c) : rows(r), cols(c) {
data.resize(rows, std::vector<double>(cols, 0));
}
// 检查矩阵是否可以相加
bool canAdd(const Matrix& other) const {
return rows == other.rows && cols == other.cols;
}
// 重载加法运算符
Matrix operator+(const Matrix& other) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 其他操作如获取元素、设置元素等...
};
```
现在你可以创建两个`Matrix`对象`a`和`b`,然后通过`+`运算符进行相加:
```cpp
Matrix a(2, 3); // 初始化第一个矩阵a
a.data = {{1, 2, 3}, {4, 5, 6}}; // 设置a的值
Matrix b(2, 3); // 初始化第二个矩阵b
b.data = {{7, 8, 9}, {10, 11, 12}}; // 设置b的值
Matrix c = a + b; // 相加,结果存储在c中
```
阅读全文
相关推荐
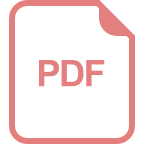















