给定一个.txt文件,使用python编写代码读取txt文件内容
时间: 2024-05-20 17:08:43 浏览: 114
当我们使用 Python 读取 .txt 文件时,可以使用内置函数 open() 来打开文件,然后使用 read() 或 readlines() 方法来读取文件内容。具体实现方法如下:
```python
# 打开文件
with open('file.txt', 'r') as f:
# 读取整个文件内容
content = f.read()
# 输出内容
print(content)
# 打开文件
with open('file.txt', 'r') as f:
# 逐行读取文件内容
lines = f.readlines()
# 输出每行内容
for line in lines:
print(line)
```
需要注意的是,使用完文件后,应该及时关闭文件,避免资源浪费和数据丢失。为了保证文件的正确关闭,可以使用 with 语句来打开文件,这样当 with 代码块执行完毕后,Python 会自动关闭文件。
相关问题
编写一个python程序:在给定的“exmaple.txt”文件中读取数据,将不是以字符“#”开头的行,保存到新文件“new_file.txt”中。 1.打开“exmaple.txt”文件。 2.读取其中的信息: (1)如果以字符“#”开头的行,不做任何操作; (2)如果不是以字符“#”开头的行,写入到新文件“new_file.txt”中。
可以尝试以下代码:
```python
with open("exmaple.txt", "r") as f:
lines = f.readlines()
with open("new_file.txt", "w") as f:
for line in lines:
if line.startswith("#"):
continue
f.write(line)
```
首先,使用 `with open()` 打开 `exmaple.txt` 文件,并将其读取到 `lines` 列表中。
然后,使用 `with open()` 打开 `new_file.txt` 文件,并遍历 `lines` 列表中的每一行,如果以字符“#”开头,则跳过;否则,将该行写入到 `new_file.txt` 文件中。
最后,使用 `with` 语句可以确保在处理文件后自动关闭文件,而不需要手动关闭。
用Python编写程序,合并多个给定的.docx文件内容为一个.docx文件,并保持原来多个文件内容的格式
在Python中,可以使用`python-docx`库来操作`.docx`文件。为了将多个`.docx`文件的内容合并到一个新的文件中,我们需要先安装`python-docx`,然后读取每个源文件,逐页添加到目标文档中。这里是一个简化的示例:
首先,安装`python-docx`库:
```bash
pip install python-docx
```
接下来,编写Python代码:
```python
from docx import Document
def merge_docx_files(input_files, output_file):
doc = Document() # 创建一个新的Word文档
for input_file in input_files:
with open(input_file, 'rb') as f:
doc.add_paragraph(f.read().decode('utf-8')) # 将源文件内容添加到新文档
doc.save(output_file) # 保存合并后的文档
# 示例:输入文件列表和输出文件路径
input_files = ['file1.docx', 'file2.docx', 'file3.docx']
output_file = 'merged.docx'
merge_docx_files(input_files, output_file)
#
阅读全文
相关推荐
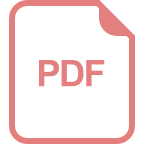
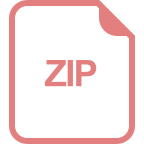









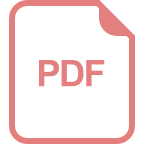